10 Essential Features of the Go Programming Language
- With Code Example
- September 5, 2024
Unlocking the Power of Go: A Developer's Perspective
As a software developer with over a decade of experience across multiple programming languages, I’ve found Go to be a game-changer in many projects. Today, I want to share my insights on what makes the Go programming language stand out from the crowd, complete with detailed explanations and code examples.
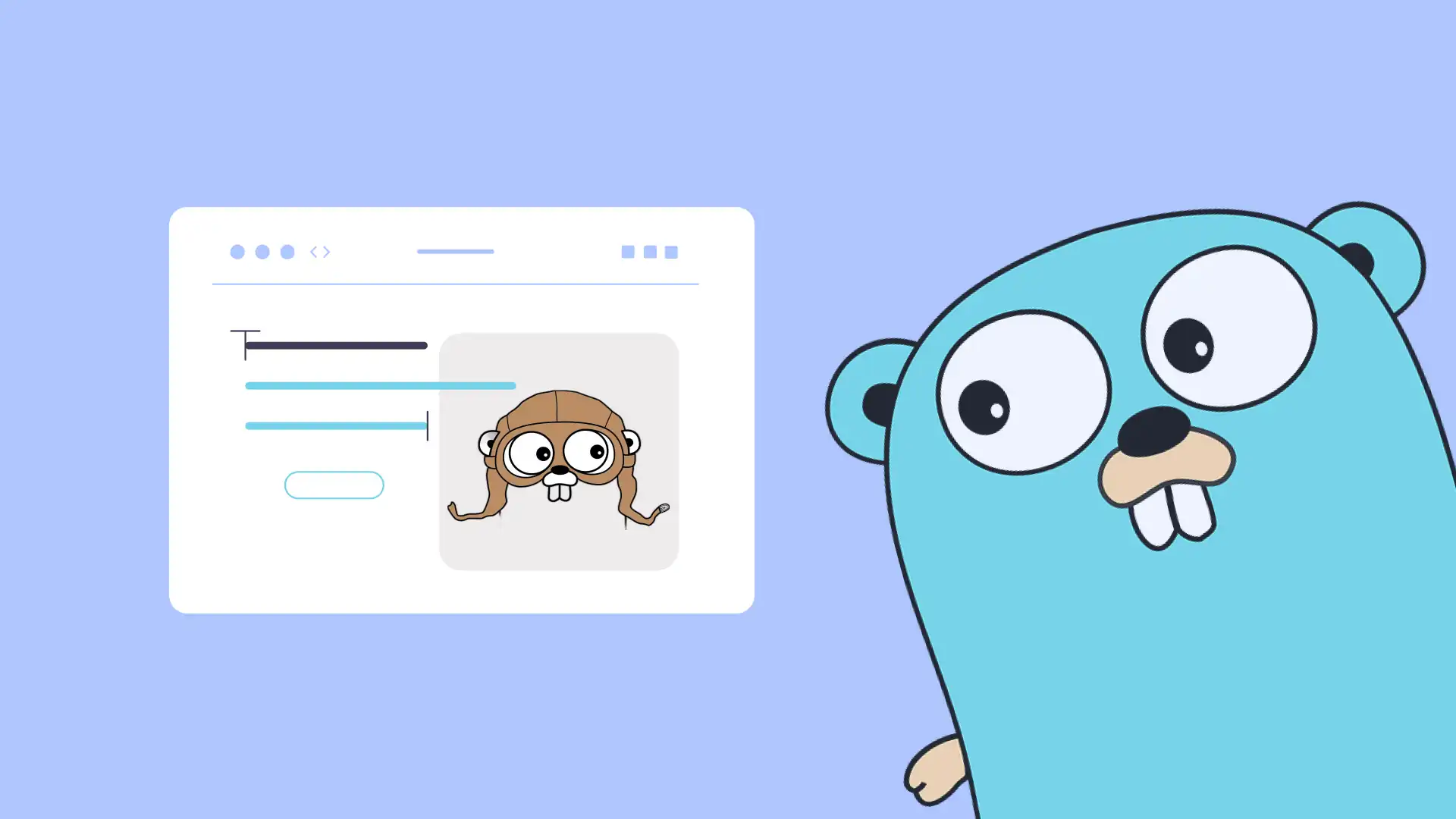
1. Simplicity and Readability
One of Go’s strongest suits is its simplicity. The language’s designers made deliberate choices to keep the syntax clean and straightforward. This makes Go code incredibly readable, even for developers new to the language.
Go achieves this simplicity through several means:
- A small set of keywords (25 in total)
- Consistent and predictable syntax
- No classes or inheritance, favoring composition instead
- No exceptions, using explicit error handling
Here’s a simple example that demonstrates Go’s readability:
package main
import "fmt"
func greet(name string) string {
return fmt.Sprintf("Hello, %s!", name)
}
func main() {
message := greet("Gopher")
fmt.Println(message)
}
In my experience, this simplicity has significantly reduced onboarding time for new team members and made code reviews a breeze.
2. Fast Compilation
Go’s compilation speed is nothing short of impressive. I’ve worked on large-scale projects where compile times in other languages were a major bottleneck. With Go, the near-instantaneous compilation has been a massive productivity boost, allowing for rapid iteration and testing.
The fast compilation is due to several factors:
- Efficient dependency analysis
- No header files, which simplifies the build process
- The compiler is designed for speed from the ground up
To demonstrate this, try compiling a simple Go program and compare it to a similar program in C++ or Java. The difference is often stark, especially as projects grow in size.
3. Built-in Concurrency
Go’s approach to concurrency with goroutines and channels is a standout feature. I’ve found it much easier to write and reason about concurrent code in Go compared to other languages.
Here’s a simple example of concurrent execution in Go:
package main
import (
"fmt"
"time"
)
func worker(id int, jobs <-chan int, results chan<- int) {
for j := range jobs {
fmt.Printf("Worker %d started job %d\n", id, j)
time.Sleep(time.Second) // Simulate work
fmt.Printf("Worker %d finished job %d\n", id, j)
results <- j * 2
}
}
func main() {
jobs := make(chan int, 100)
results := make(chan int, 100)
for w := 1; w <= 3; w++ {
go worker(w, jobs, results)
}
for j := 1; j <= 5; j++ {
jobs <- j
}
close(jobs)
for a := 1; a <= 5; a++ {
<-results
}
}
This example demonstrates how easy it is to spin up multiple workers and coordinate their work using channels. This has been particularly useful in developing high-performance, scalable backend services.
4. Strong Standard Library
The Go standard library is comprehensive and well-designed. In many cases, I’ve been able to build robust applications with minimal third-party dependencies, which has simplified deployment and reduced potential security risks.
Some standout packages in the standard library include:
net/http
for HTTP clients and serversencoding/json
for JSON parsing and generationdatabase/sql
for database operations
Here’s an example of a simple HTTP server using just the standard library:
package main
import (
"fmt"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello, %s!", r.URL.Path[1:])
}
func main() {
http.HandleFunc("/", handler)
http.ListenAndServe(":8080", nil)
}
This powerful functionality out of the box has often saved me time and reduced the complexity of my projects.
5. Cross-Platform Support
Go’s ability to easily cross-compile for different platforms has been a game-changer for my team. We can develop on our local machines and deploy to various server environments without headaches.
To cross-compile, you simply set the GOOS
and GOARCH
environment variables before building:
GOOS=linux GOARCH=amd64 go build main.go
This command compiles the program for Linux on a 64-bit AMD architecture, regardless of your development machine’s OS.
6. Garbage Collection
While some may see garbage collection as a performance hit, Go’s implementation is efficient and unobtrusive. In my projects, it has eliminated a whole class of memory management bugs without noticeably impacting performance.
Go’s garbage collector is concurrent, which means it can run simultaneously with the main program, reducing pause times. It’s also generational, focusing on newer objects first, which tend to have shorter lifetimes.
While you don’t interact directly with the garbage collector in your code, you can tune its behavior if needed:
import "runtime"
func main() {
runtime.GOMAXPROCS(4) // Set the number of CPUs to use
runtime.GC() // Force a garbage collection
}
7. Interface-Based Design
Go’s interface system is both powerful and flexible. I’ve found it encourages good design practices and makes it easy to write testable, modular code. The implicit interface implementation has led to more decoupled and maintainable codebases in my experience.
Here’s an example of how interfaces work in Go:
package main
import "fmt"
type Writer interface {
Write([]byte) (int, error)
}
type ConsoleWriter struct{}
func (cw ConsoleWriter) Write(data []byte) (int, error) {
n, err := fmt.Println(string(data))
return n, err
}
func main() {
var w Writer = ConsoleWriter{}
w.Write([]byte("Hello, Interface!"))
}
This design allows for easy mocking in tests and swapping implementations without changing the using code.
8. Tooling Support
The Go ecosystem comes with excellent built-in tools. The go
command for building, testing, and managing dependencies, along with tools like gofmt
for consistent code formatting, have significantly improved my development workflow.
Some key tools include:
go fmt
for automatic code formattinggo test
for running testsgo vet
for detecting subtle bugsgo mod
for dependency management
Here’s how you might use some of these tools:
go fmt ./... # Format all Go files in the current directory and subdirectories
go test ./... # Run all tests
go vet ./... # Run the vet tool on all packages
9. Error Handling
While controversial to some, I’ve grown to appreciate Go’s explicit error handling. It forces developers to think about and handle error cases, leading to more robust and reliable software in production environments.
Here’s an example of typical error handling in Go:
package main
import (
"fmt"
"os"
)
func readFile(filename string) error {
file, err := os.Open(filename)
if err != nil {
return fmt.Errorf("error opening file: %w", err)
}
defer file.Close()
// Read file contents...
return nil
}
func main() {
err := readFile("nonexistent.txt")
if err != nil {
fmt.Println("An error occurred:", err)
return
}
fmt.Println("File read successfully")
}
This approach ensures that errors are dealt with explicitly, reducing the chance of overlooked failure cases.
10. Growing Ecosystem
Although younger than some other languages, Go’s ecosystem is thriving. I’ve seen a steady growth in high-quality libraries and frameworks, making it easier to find tools and solutions for various development needs.
Some popular third-party libraries include:
- Gin for web applications
- GORM for object-relational mapping
- Cobra for creating powerful CLI applications
Here’s a quick example using Gin:
package main
import "github.com/gin-gonic/gin"
func main() {
r := gin.Default()
r.GET("/ping", func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "pong",
})
})
r.Run() // listen and serve on 0.0.0.0:8080
}
Conclusion
The Go programming language has become an essential tool in my development arsenal. Its focus on simplicity, performance, and practicality has made it my go-to choice for many projects, especially in the realm of backend and systems programming.
While it may not be the perfect fit for every scenario, understanding these key features of Go can help you make informed decisions about when and where to use it in your own projects. As with any technology, I encourage you to dive in, experiment, and see how Go can enhance your development process.
The examples provided here only scratch the surface of what’s possible with Go. As you explore further, you’ll discover even more powerful features and idioms that make Go a joy to work with.