Implementing NoSQL with Golang- A Step-by-Step Guide
- With Code Example
- April 30, 2024
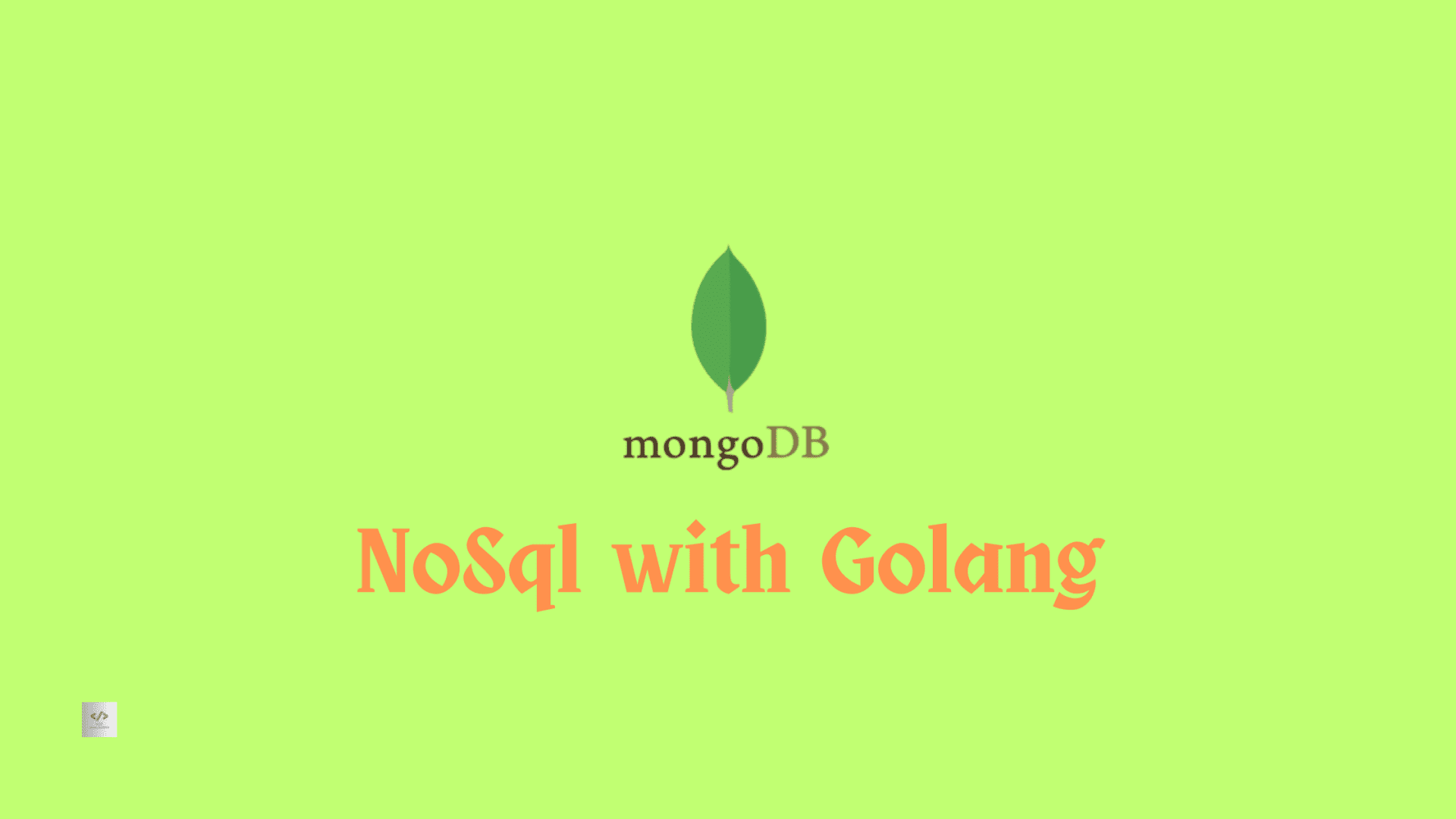
Series - golang with
Among the essentials of software engineering of the present age, there are two considerable qualities: adaptability and scalability. Enters NoSQL databasesand Golang, a dynamic duo which is on their way to change the world of developmentperpetually. Starting from a NoSQL architecture, without any schema design and supporting horizontal scalability, this gives maximum flexibility with regards to a broad variety of data types. Partnership of the straightforwardness and swiftness of Golang gives the developer enough confidence to produce excellent applications without unnecessary toil. Here we go through a comprehensive guide where we embark on a journey to discover an easier way of mixing NoSQL databases with Golang, this will be done through each step explained with clarity and also instruction by code examples.
Introduction to NoSQL:
The adoption of noSQL databases which not suprisingly are called for “Not Only SQL” as an alternative solution to traditional relational databases has gradually become widespread. Unlike their SQL counterparts, NoSQL databases have abandoned the use of rigid schemas, and named “flexible data models” as the reasons why they have done so, making it obvious that they are a perfect match for structured data only. MongoDB, KEY-VALUE stores in the NoSQL data store system are differentiated by their specialized and independent options that are created to suit the wide range demands of different applications. Some NoSQL features have been known in aiding with horizontal scalability, high availability and real-time analytics. Such features render developers to overcome modern challenges, equipping them with agility and efficiency.
Install MongoDB with Docker Compose:
Let’s kickstart our journey by setting up MongoDB effortlessly using Docker Compose. Below is a sample docker-compose.yml
file to get you started:
version: '3'
services:
mongodb:
image: mongo:latest
restart: always
environment:
MONGO_INITDB_ROOT_USERNAME: your_username
MONGO_INITDB_ROOT_PASSWORD: your_password
ports:
- "27017:27017"
volumes:
- mongodb-data:/data/db
volumes:
mongodb-data:
This YAML configuration defines a MongoDB service with your specified credentials and exposes the default MongoDB port (27017). By running docker-compose up -d
, Docker Compose will spin up the MongoDB container, ready for your Golang application to connect.
Install Golang Package for MongoDB:
To seamlessly interact with MongoDB in your Golang application, you’ll need the mongo-go-driver
package, the official MongoDB driver for Golang. Installation is straightforward with Go modules:
go get go.mongodb.org/mongo-driver/mongo
Once installed, you’re all set to harness the power of MongoDB within your Golang codebase.
Create Connection:
Establishing a connection between your Golang application and MongoDB database is the first step to data interaction. Here’s how you can create a connection in Golang:
package main
import (
"context"
"fmt"
"log"
"time"
"go.mongodb.org/mongo-driver/mongo"
"go.mongodb.org/mongo-driver/mongo/options"
)
func main() {
client, err := mongo.NewClient(options.Client().ApplyURI("mongodb://your_username:your_password@localhost:27017"))
if err != nil {
log.Fatal(err)
}
ctx, cancel := context.WithTimeout(context.Background(), 10*time.Second)
defer cancel()
err = client.Connect(ctx)
if err != nil {
log.Fatal(err)
}
defer client.Disconnect(ctx)
fmt.Println("Successfully connected to MongoDB!")
}
Replace your_username
and your_password
with your MongoDB credentials. This code snippet establishes a connection to your MongoDB database and verifies its accessibility.
Insert Data:
Adding data to your MongoDB database from a Golang application is a breeze. Consider the following example:
collection := client.Database("your_database").Collection("your_collection")
_, err := collection.InsertOne(ctx, bson.D{{"name", "John Doe"}, {"age", 30}})
if err != nil {
log.Fatal(err)
}
fmt.Println("Data inserted successfully!")
This snippet inserts a document into the specified collection in your MongoDB database.
Update Data:
Updating existing documents in your MongoDB database via Golang is straightforward. Take a look:
collection := client.Database("your_database").Collection("your_collection")
filter := bson.D{{"name", "John Doe"}}
update := bson.D{{"$set", bson.D{{"age", 31}}}}
_, err := collection.UpdateOne(ctx, filter, update)
if err != nil {
log.Fatal(err)
}
fmt.Println("Data updated successfully!")
This code updates the age of a document with the name “John Doe” in the specified collection.
Select Data:
Retrieving data from MongoDB collections in your Golang application is as simple as it gets. Here’s a quick example:
collection := client.Database("your_database").Collection("your_collection")
cursor, err := collection.Find(ctx, bson.D{})
if err != nil {
log.Fatal(err)
}
defer cursor.Close(ctx)
for cursor.Next(ctx) {
var result bson.M
err := cursor.Decode(&result)
if err != nil {
log.Fatal(err)
}
fmt.Println(result)
}
if err := cursor.Err(); err != nil {
log.Fatal(err)
}
This snippet fetches all documents from the specified collection in your MongoDB database and prints them out.
Delete Data:
Removing unwanted documents from your MongoDB database via Golang is straightforward. Here’s an example:
collection := client.Database("your_database").Collection("your_collection")
filter := bson.D{{"name", "John Doe"}}
_, err := collection.DeleteOne(ctx, filter)
if err != nil {
log.Fatal(err)
}
fmt.Println("Data deleted successfully!")
Conclusion:
A review shows that combining MongoDB and Golang into one platform enables the developers to build apps that are dynamic and scalable. Because of the the-ability of NoSQL data schema and the speed of Go, you simply need to solve modern day problem. The programming code for each step is supplied with detailed illustration of its practical application that is aimed ac facilitating your development workflow. Try to learn about the fusion of NoSQL and Golang languages during your projects and know exceptional opportunities which will increase in your software work.