Chapter 9: Deploying Gin Applications
- With Code Example
- July 21, 2024
Deploying Gin Applications: A Step-by-Step Guide
Series - GIN Course
- 1: Chapter-1: Introduction to Gin Framework
- 2: Chapter 2: Setting Up the Environment for GIN Framework
- 3: Chapter 3: Project Structure In Gin Framework
- 4: Chapter 4: Getting Started with Gin
- 5: Chapter 5: Working with Models and Databases
- 6: Chapter 6: Advanced Routing and URL Parameters in Gin
- 7: Chapter 7: Authentication And Authorization In Gin
- 8: Chapter 8: Testing and Debugging in Gin
- 9: Chapter 9: Deploying Gin Applications
- 10: Chapter 10: Real-world Projects and Best Practices with Gin
In this chapter, we’ll explore the steps involved in preparing your Gin application for deployment, different deployment options, and setting up Continuous Integration/Continuous Deployment (CI/CD) pipelines. This includes managing environment variables, deploying to platforms like Heroku and cloud providers such as AWS, GCP, or Azure, and automating deployments with CI/CD tools.
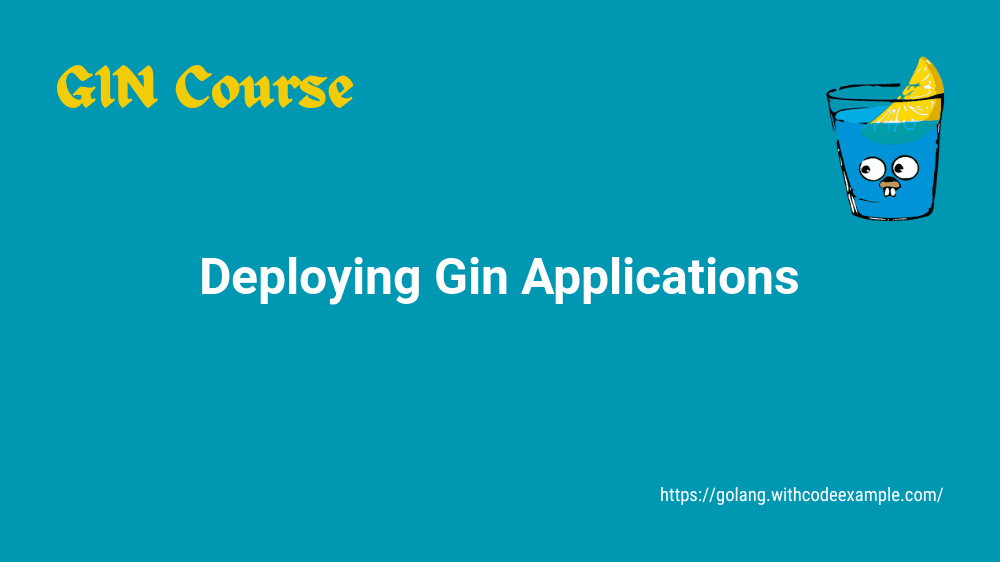
Preparing Your Application for Deployment
Environment Variables
Managing environment variables is crucial for configuring your application based on different environments (development, testing, production). Use environment variables to store sensitive data such as database credentials, API keys, and other configuration settings.
Example: Using Environment Variables
- Create a
.env
file to store your environment variables:
PORT=8080
DATABASE_URL=postgres://user:password@localhost:5432/dbname
JWT_SECRET=mysecretkey
- Use a package like
github.com/joho/godotenv
to load these variables into your application:
package main
import (
"log"
"os"
"github.com/gin-gonic/gin"
"github.com/joho/godotenv"
)
func init() {
err := godotenv.Load()
if err != nil {
log.Fatal("Error loading .env file")
}
}
func main() {
r := gin.Default()
port := os.Getenv("PORT")
if port == "" {
port = "8080"
}
r.GET("/ping", func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "pong",
})
})
r.Run(":" + port)
}
Configuration Management
It’s important to have a structured way of managing configuration settings. Use a configuration file (e.g., JSON, YAML) to store settings and load them into your application.
Example: Configuration Management
- Create a
config.json
file:
{
"port": "8080",
"database_url": "postgres://user:password@localhost:5432/dbname",
"jwt_secret": "mysecretkey"
}
- Load the configuration in your application:
package main
import (
"encoding/json"
"log"
"os"
"github.com/gin-gonic/gin"
)
type Config struct {
Port string `json:"port"`
DatabaseURL string `json:"database_url"`
JWTSecret string `json:"jwt_secret"`
}
func loadConfig() (*Config, error) {
file, err := os.Open("config.json")
if err != nil {
return nil, err
}
defer file.Close()
config := &Config{}
decoder := json.NewDecoder(file)
err = decoder.Decode(config)
if err != nil {
return nil, err
}
return config, nil
}
func main() {
config, err := loadConfig()
if err != nil {
log.Fatal("Error loading configuration: ", err)
}
r := gin.Default()
r.GET("/ping", func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "pong",
})
})
r.Run(":" + config.Port)
}
Deployment Options
Deploying to Heroku
Heroku is a popular platform-as-a-service (PaaS) that simplifies the deployment process.
Steps to Deploy to Heroku
- Create a
Procfile
in the root directory of your project:
web: ./your_gin_app
- Create a Heroku app and deploy:
heroku create your-app-name
git push heroku main
- Set environment variables on Heroku:
heroku config:set PORT=8080 DATABASE_URL=your_database_url JWT_SECRET=your_secret_key
- Open your app:
heroku open
Deploying to AWS, GCP, or Azure
AWS
Elastic Beanstalk is a great choice for deploying Gin applications.
Docker: Create a
Dockerfile
:
FROM golang:1.16-alpine
WORKDIR /app
COPY . .
RUN go build -o main .
CMD ["./main"]
- Deploy using Elastic Beanstalk CLI:
eb init -p docker your-app-name
eb create your-environment-name
eb deploy
GCP
- Google App Engine: Create an
app.yaml
:
runtime: go116
- Deploy using gcloud CLI:
gcloud app deploy
Azure
Azure App Service: Use the Azure CLI to create and deploy your application.
Deploy using Azure CLI:
az webapp up --name your-app-name --resource-group your-resource-group --plan your-app-service-plan
CI/CD for Gin Applications
Setting up Continuous Integration
Continuous Integration (CI) involves automatically running tests and building your application whenever changes are pushed to your repository.
Example: Setting up CI with GitHub Actions
- Create a
.github/workflows/ci.yml
file:
name: CI
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Go
uses: actions/setup-go@v2
with:
go-version: 1.16
- name: Install dependencies
run: go mod tidy
- name: Run tests
run: go test ./...
Automating Deployments
Automating deployments ensures that your application is deployed whenever changes are merged into the main branch.
Example: Automating Deployments with GitHub Actions
- Extend the CI workflow to include deployment:
name: CI/CD
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Go
uses: actions/setup-go@v2
with:
go-version: 1.16
- name: Install dependencies
run: go mod tidy
- name: Run tests
run: go test ./...
- name: Deploy to Heroku
env:
HEROKU_API_KEY: ${{ secrets.HEROKU_API_KEY }}
run: |
git remote add heroku https://git.heroku.com/your-app-name.git
git push heroku main
In this workflow, the application is built, tested, and deployed to Heroku whenever changes are pushed to the main branch.
By following the practices outlined in this chapter, you can effectively prepare, deploy, and manage your Gin applications with a robust CI/CD pipeline. Happy deploying!