Chapter 5: Working with Models and Databases
- With Code Example
- March 8, 2024
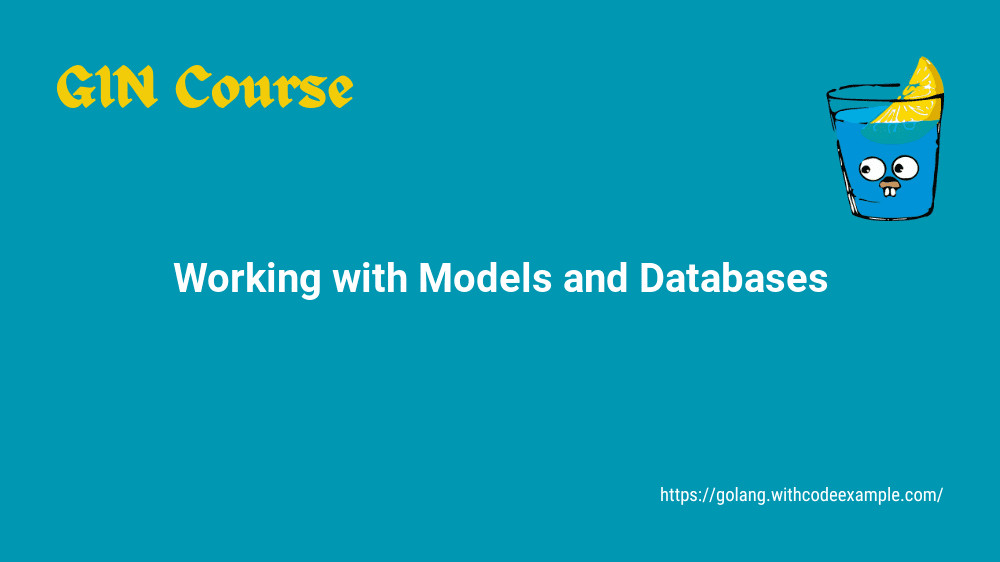
Series - GIN Course
- 1: Chapter-1: Introduction to Gin Framework
- 2: Chapter 2: Setting Up the Environment for GIN Framework
- 3: Chapter 3: Project Structure In Gin Framework
- 4: Chapter 4: Getting Started with Gin
- 5: Chapter 5: Working with Models and Databases
- 6: Chapter 6: Advanced Routing and URL Parameters in Gin
- 7: Chapter 7: Authentication And Authorization In Gin
- 8: Chapter 8: Testing and Debugging in Gin
- 9: Chapter 9: Deploying Gin Applications
- 10: Chapter 10: Real-world Projects and Best Practices with Gin
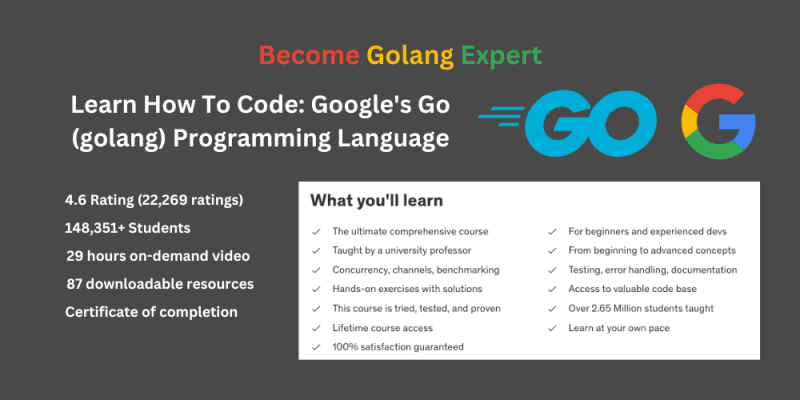
In this blog of Gin tutorial , we will explore how to seamlessly integrate models and databases with the Gin framework, enabling you to build robust and scalable web applications. By leveraging popular libraries and following best practices, you’ll learn how to define models, establish database connections, perform CRUD operations, and ensure data integrity within your Gin-based projects.
Table of Contents
Integrating with Databases
Connecting to a Database with Gin
Gin keeps things lightweight and simple, but don’t let that fool you – it’s incredibly powerful when it comes to handling data. By leveraging the right database drivers and libraries, you can connect to popular databases like PostgreSQL, MySQL, or SQLite with just a few lines of code. And the best part? Gin lets you configure your database settings right from the start, so you can hit the ground running and focus on building amazing features instead of wrestling with complex setup procedures. In this guide, we’ll walk through the whole process step-by-step, showing you how to define your models, connect to your database, and perform all the essential CRUD operations you need to build a rock-solid web app. So, let’s dive in and unlock the true power of Gin!
Let’s illustrate this with an example using PostgreSQL:
package main
import (
"github.com/gin-gonic/gin"
"gorm.io/driver/postgres"
"gorm.io/gorm"
)
func main() {
r := gin.Default()
// Initialize PostgreSQL connection
dsn := "user=your_user password=your_password dbname=your_db sslmode=disable"
db, err := gorm.Open(postgres.Open(dsn), &gorm.Config{})
if err != nil {
panic("failed to connect database")
}
// Register routes and handlers
// ...
r.Run(":8080")
}
Using ORM with GORM
One of the best parts about working with Gin is how seamlessly it integrates with powerful Object-Relational Mapping (ORM) tools like GORM . This magical combination takes a lot of the headache out of interacting with databases. Instead of getting bogged down in the nitty-gritty details of database management, GORM lets you work at a higher level of abstraction. Want to map a Go struct directly to a database table? GORM’s got you covered. Need to handle migrations automatically? No problem. Building complex queries? GORM makes it a breeze. By taking care of all these low-level tasks for you, the dynamic duo of Gin and GORM frees you up to focus on the real meat of your application logic. It’s a huge productivity boost and a real game-changer for maintaining clean, readable code over the long haul. Trust me, once you experience the power of this integration, you’ll never want to go back to the old way of doing things.
GORM simplifies CRUD operations and object-relational mapping. Here’s how to integrate GORM with Gin:
type User struct {
gorm.Model
Name string
Email string `gorm:"uniqueIndex"`
}
func main() {
r := gin.Default()
// Initialize GORM with PostgreSQL
db, err := gorm.Open(postgres.Open(dsn), &gorm.Config{})
if err != nil {
panic("failed to connect database")
}
// Migrate the schema
db.AutoMigrate(&User{})
// Register routes and handlers
// ...
r.Run(":8080")
}
Performing CRUD Operations on Models
One of the real strengths of Gin is its elegant routing system, which makes implementing those essential CRUD (Create, Read, Update, Delete) operations on your models an absolute breeze. By defining a set of RESTful endpoints and associating each one with a corresponding handler function, you can build out all the functionality you need to manage your model data with minimal fuss.
But Gin’s routing capabilities are just the start. Thanks to its flexible middleware system, you can easily layer in additional features like authentication, authorization, and input validation. Simply put, Gin gives you all the tools you need to ensure your data stays secure and maintains integrity, without tying you up in complex implementations.
The real beauty here is how seamlessly everything comes together. You can quickly set up your routes, define your handlers, and plug in the necessary middleware – all while keeping your code clean, readable, and maintainable. It’s a developer’s dream come true!
So, whether you’re building a simple CRUD app or a more complex system with advanced data management needs, Gin’s got your back. With its powerful routing and middleware support, you can focus on writing awesome code and delivering killer features, knowing that your data is in good hands.
func main() {
// Initialize Gin and GORM
r.GET("/users", func(c *gin.Context) {
var users []User
db.Find(&users)
c.JSON(200, users)
})
r.POST("/users", func(c *gin.Context) {
var user User
if err := c.ShouldBindJSON(&user); err != nil {
c.JSON(400, gin.H{"error": err.Error()})
return
}
db.Create(&user)
c.JSON(201, user)
})
// Implement other CRUD operations (GET, PUT, DELETE)
// ...
r.Run(":8080")
}
Data Validation and Serialization
Validating Incoming Data
When it comes to building rock-solid web applications, data validation is absolutely critical. After all, the last thing you want is garbage data sneaking into your system and causing all sorts of headaches down the line. Luckily, Gin has your back with its powerful validation middleware.
This handy feature allows you to define a set of validation rules for all incoming requests, ensuring that any data coming into your application meets your specific criteria before it gets processed. It’s like having a bouncer at the door, keeping out any unwanted guests (or in this case, invalid data).
The best part? Setting up validation rules is a breeze. All you have to do is annotate your request structs with special validation tags, like binding:"required"
. Gin will automatically check incoming data against these tags, and if anything doesn’t meet the requirements, it’ll get bounced before it can cause any trouble.
Talk about peace of mind! With Gin’s validation middleware in place, you can trust that your application is only dealing with clean, valid data – no more worrying about garbage input causing bugs, crashes, or security vulnerabilities. It’s just one more way that Gin helps streamline the development process and keeps your code safe, secure, and maintainable.
Gin’s validation middleware simplifies data validation:
type CreateUserRequest struct {
Name string `json:"name" binding:"required"`
Email string `json:"email" binding:"required,email"`
}
func main() {
r.POST("/users", func(c *gin.Context) {
var req CreateUserRequest
if err := c.ShouldBindJSON(&req); err != nil {
c.JSON(400, gin.H{"error": err.Error()})
return
}
// Process valid request
c.JSON(201, gin.H{"message": "User created successfully"})
})
// Other routes and handlers
}
Serializing and Deserializing JSON Data
No more wrestling with complex third-party libraries or getting bogged down in tedious boilerplate code. Gin’s JSON handling is as straightforward and elegant as it gets, allowing you to focus your energy on building killer features instead of sweating the small stuff.
Whether you’re crafting a RESTful API, integrating with external services, or simply passing data back and forth between client and server, Gin’s JSON capabilities have got you covered. It’s just one more example of how this fantastic framework streamlines the development process and empowers you to build amazing applications with minimal fuss.
So, if you’re working in Go and dealing with JSON data (and let’s be honest, who isn’t these days?), make sure to take full advantage of Gin’s JSON superpowers. It’s a game-changer for productivity, maintainability, and overall developer happiness.
func main() {
type UserResponse struct {
ID uint `json:"id"`
Name string `json:"name"`
Email string `json:"email"`
}
r.GET("/users/:id", func(c *gin.Context) {
var user User
if err := db.First(&user, c.Param("id")).Error; err != nil {
c.JSON(404, gin.H{"error": "User not found"})
return
}
// Serialize user data
userResp := UserResponse{ID: user.ID, Name: user.Name, Email: user.Email}
c.JSON(200, userResp)
})
// Other routes and handlers
}
Handling Form Submissions
Handling form submissions is a common task in web development, and Gin streamlines this process with its Form
and Bind
methods. Developers can easily bind form data to Go structs, enabling seamless processing and validation. Moreover, Gin’s middleware ecosystem allows for the integration of CSRF protection, enhancing application security without added complexity.
type CreateUserForm struct {
Name string `form:"name" binding:"required"`
Email string `form:"email" binding:"required,email"`
}
func main() {
r.POST("/users", func(c *gin.Context) {
var form CreateUserForm
if err := c.ShouldBind(&form); err != nil {
c.JSON(400, gin.H{"error": err.Error()})
return
}
// Process valid form submission
c.JSON(201, gin.H{"message": "User created successfully"})
})
// Other routes and handlers
}
Conclusion
The Gin framework, combined with GORM, provides a powerful solution for managing models and databases in Go applications. By leveraging GORM’s ORM capabilities seamlessly integrated into Gin, developers can work at a higher level of abstraction, abstracting away low-level database details. Gin’s elegant routing system streamlines CRUD operations, while its middleware ecosystem enables seamless integration of essential features like input validation and CSRF protection. Understanding and implementing these concepts empowers developers to create scalable, efficient, and secure web applications with minimal effort. Explore further by referring to the Gin documentation and GORM documentation .
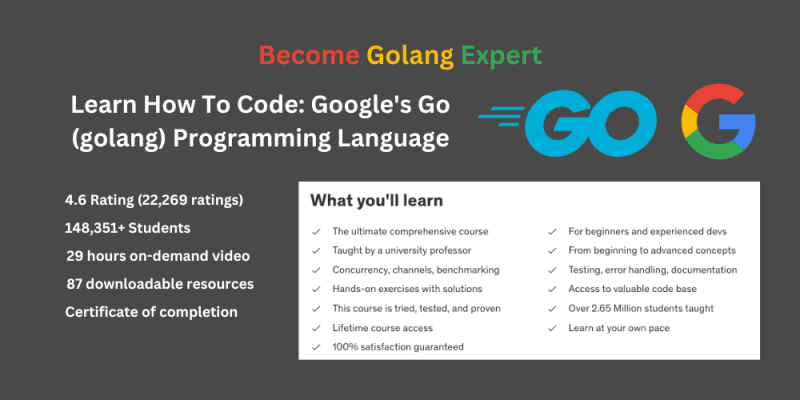