Generics in GoLang: A Guide to Better Code Reuse
- With Code Example
- May 14, 2024
Reuse Your Code Like a Pro: The Power of Generics in GoLang
Generics have been a much-anticipated feature for Go developers, providing a means to write more flexible and reusable code. Generics allow functions, data structures, and types to operate with any data type while still benefiting from Go’s static typing and performance advantages. Released in Go 1.18, generics have opened up a wealth of opportunities for developers to simplify and optimize their code.
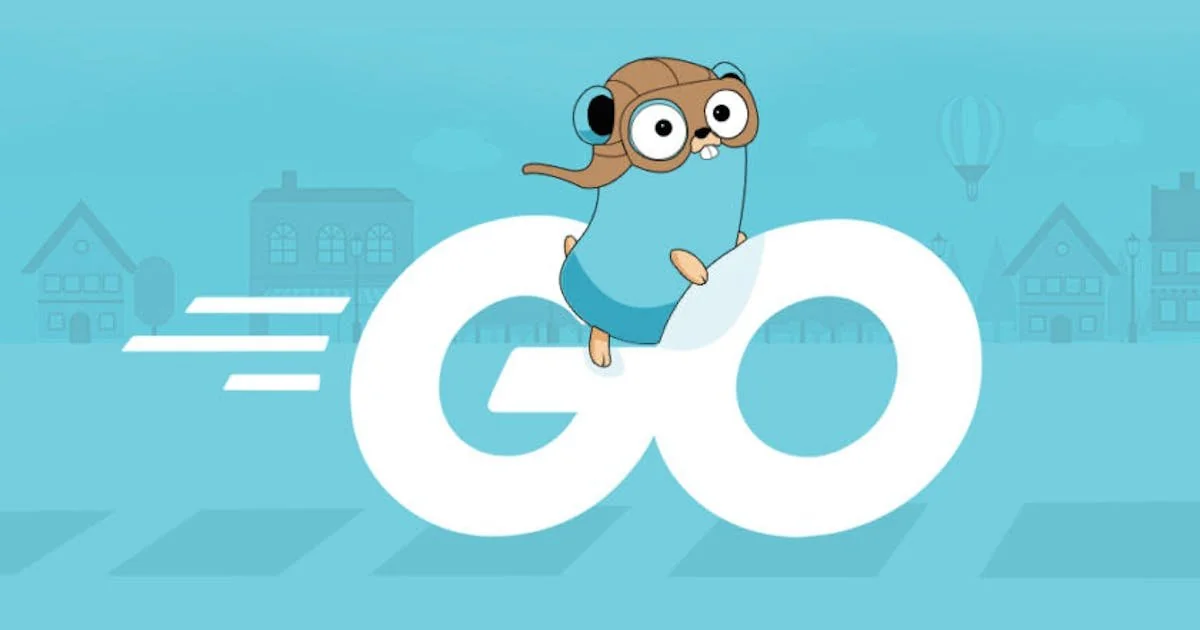
In this article, we will dive deep into Go generics, exploring their syntax, usage, and practicality through illustrative code examples.
Table of Contents
What are Generics?
Generics allow you to define algorithms and data structures in a way that can operate on any data type. In traditional Go (prior to version 1.18), if you needed a function or data structure to operate on multiple types, you would have to:
- Use interfaces, which can lead to type assertions and potential runtime errors.
- Write multiple versions of the same function or data structure for each type, leading to redundancy.
Generics eliminate these issues by enabling type parameters that are specified when a function or type is declared.
Basic Syntax
The syntax for generics in Go involves the use of type parameters. Here’s a simple generic function example:
package main
import "fmt"
// Swap is a generic function that swaps the values of two variables
func Swap[T any](a, b T) (T, T) {
return b, a
}
func main() {
x, y := 3, 4
fmt.Println("Before swap:", x, y)
x, y = Swap(x, y)
fmt.Println("After swap:", x, y)
a, b := "hello", "world"
fmt.Println("Before swap:", a, b)
a, b = Swap(a, b)
fmt.Println("After swap:", a, b)
}
In this example:
func Swap[T any](a, b T) (T, T)
declares a generic functionSwap
.[T any]
specifies a type parameterT
which can be of any type (any
is a built-in constraint that allows any type).- The function can now accept parameters of any type and return values of the same type.
Generic Data Structures
Generics are also extremely useful for data structures like lists, trees, and more. Let’s create a generic stack:
package main
import "fmt"
// Stack is a generic stack
type Stack[T any] struct {
elements []T
}
// Push adds an element to the stack
func (s *Stack[T]) Push(element T) {
s.elements = append(s.elements, element)
}
// Pop removes and returns the top element of the stack
func (s *Stack[T]) Pop() (T, bool) {
if len(s.elements) == 0 {
var zeroValue T
return zeroValue, false
}
element := s.elements[len(s.elements)-1]
s.elements = s.elements[:len(s.elements)-1]
return element, true
}
func main() {
// Integer stack
intStack := Stack[int]{}
intStack.Push(1)
intStack.Push(2)
intStack.Push(3)
fmt.Println(intStack.Pop()) // Should print 3, true
fmt.Println(intStack.Pop()) // Should print 2, true
fmt.Println(intStack.Pop()) // Should print 1, true
fmt.Println(intStack.Pop()) // Should print 0, false (zero value of int)
// String stack
stringStack := Stack[string]{}
stringStack.Push("a")
stringStack.Push("b")
stringStack.Push("c")
fmt.Println(stringStack.Pop()) // Should print c, true
fmt.Println(stringStack.Pop()) // Should print b, true
fmt.Println(stringStack.Pop()) // Should print a, true
fmt.Println(stringStack.Pop()) // Should print "", false (zero value of string)
}
In this example:
type Stack[T any] struct
declares a generic stack type.Push
andPop
methods operate on elements of typeT
, allowing stacks of any element type to be managed efficiently.
Constraints
Go also allows you to enforce constraints on generic types, ensuring they satisfy specific behaviors or interfaces. For example, let’s create a function that sums numbers:
package main
import "fmt"
// Number is an interface that constraints T to be int, float64, etc.
type Number interface {
int | int64 | float64
}
// Sum adds all elements in the slice
func Sum[T Number](numbers []T) T {
var sum T
for _, number := range numbers {
sum += number
}
return sum
}
func main() {
intNumbers := []int{1, 2, 3, 4}
floatNumbers := []float64{1.1, 2.2, 3.3, 4.4}
fmt.Println(Sum(intNumbers)) // Should print 10
fmt.Println(Sum(floatNumbers)) // Should print 11.0
}
In this example:
type Number interface { ... }
defines a constraint interface that includesint
,int64
, andfloat64
.func Sum[T Number](numbers []T) T
ensures theSum
function works only with types that satisfy theNumber
constraint.
Conclusion
Generics in GoLang allow for more powerful, reusable, and type-safe code. This feature reduces redundancy and opens up new possibilities for developers to write cleaner code. As you explore and integrate generics into your Go projects, remember that while they offer great flexibility, it’s essential always to balance them with simplicity and readability to maintain Go’s ethos of clear and concise code.
Happy coding with Go generics!