Documentation and Comments in Go
- With Code Example
- August 16, 2023
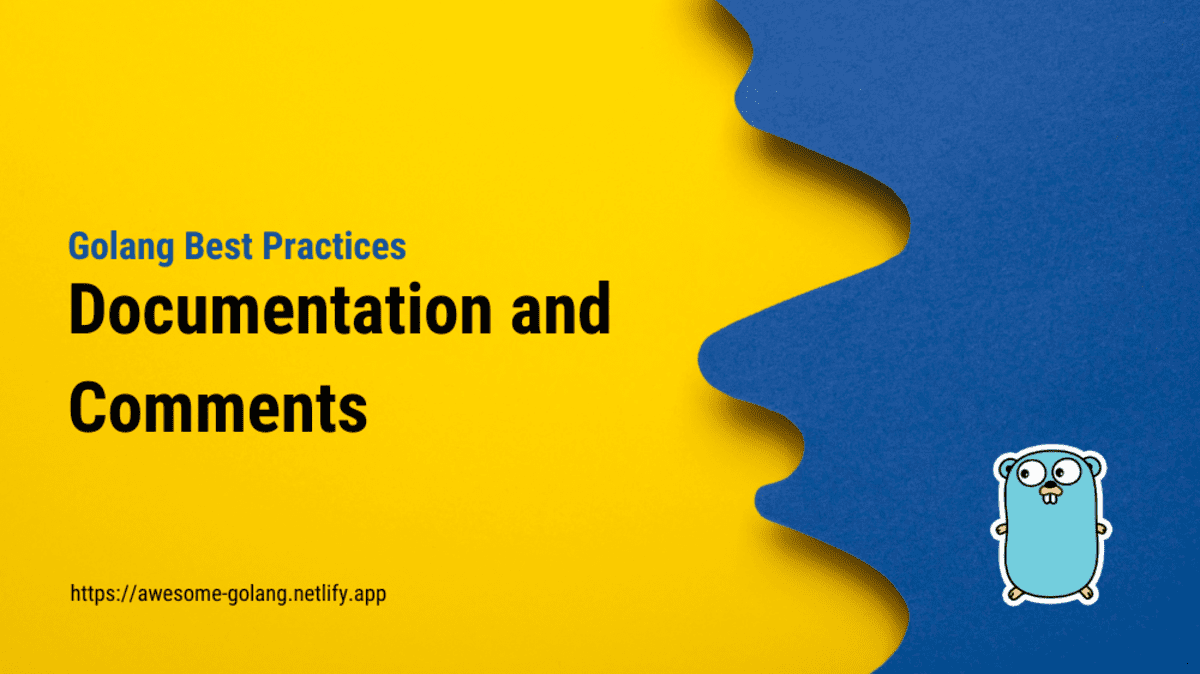
Series - Golang Best Practices
- 1: Introduction to Golang Best Practices
- 2: Code Formatting and Style - A Guide for Developers
- 3: How To Handle Errors In Golang Effectively
- 4: Concurrency and Goroutines - Learn Parallelism in Golang
- 5: Memory Management in Golang - Safeguarding Efficiency and Stability
- 6: Testing, Benchmarking and Continuous Integration in Golang
- 7: Performance Optimization in Golang
- 8: Package and Module Design in Golang
- 9: Security Best Practices for Go Applications
- 10: Documentation and Comments in Go
- 11: Debugging Techniques in Golang
- 12: Continuous Improvement and Code Reviews
- 13: Understanding Error Handing in Golang
In the realm of software development, writing code is only half the battle. The other half revolves around creating clear, concise, and comprehensive documentation that not only aids developers in understanding the codebase but also serves as a roadmap for future development. In this guide, we delve into the essential practices for writing effective code comments and harnessing the power of documentation tools like Godoc and GoDoc to ensure your Golang projects are well-documented, easily maintainable, and a joy to work on.
Writing Clear and Concise Code Comments
Code comments serve as a crucial communication channel between developers, conveying the intentions, logic, and nuances of the code. Well-crafted comments can significantly enhance code readability and maintainability.
Commenting Best Practices
- Use comments to explain the “why” behind your code, not just the “what.”
- Keep comments concise, avoiding unnecessary verbosity.
- Comment complex or non-intuitive sections of code to provide context.
- Update comments when you modify code to ensure they remain accurate.
package main
import "fmt"
func main() {
// Calculate the sum of two numbers
sum := add(5, 7)
fmt.Println("Sum:", sum)
}
// add returns the sum of two integers
func add(a, b int) int {
return a + b
}
Documenting Functions and Packages with Godoc
Godoc is a tool that automatically generates documentation for your Go code. By adhering to a simple set of conventions, you can create comprehensive documentation that’s easily accessible to both developers and users.
Godoc Conventions
- Place comments directly above function and type declarations.
- Begin the comment with the name of the function or type.
- Use full sentences to describe functionality and usage.
package main
import "fmt"
// greet prints a friendly greeting message.
func greet(name string) {
fmt.Println("Hello,", name)
}
func main() {
greet("Alice")
}
Leveraging GoDoc for Code Documentation
GoDoc is a web-based tool that presents your Godoc comments in an easily navigable format. It provides an organized and user-friendly way to explore your codebase’s documentation.
Accessing GoDoc Documentation
- Import the package you want to document in your Go code.
- Use the
go doc
command to view the documentation.
$ go doc fmt
$ go doc fmt.Printf
$ go doc github.com/username/project/package
Conclusion
In the world of software development, documentation isn’t just a nice-to-have – it’s a necessity. Writing clear, concise code comments and leveraging tools like Godoc and GoDoc empower you to create well-documented Golang projects that are not only easier to maintain but also more inviting for other developers to contribute to. By embracing these practices, you enrich your codebase with context, insights, and guidance, fostering collaboration and ensuring the longevity of your Golang endeavors.
Related Posts
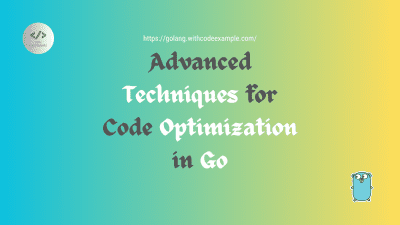
Advanced Techniques for Code Optimization in Go
Go, also known as Golang, is celebrated for its simplicity, readability, and efficiency. While the language itself encourages clean and idiomatic code, there are various advanced techniques and best practices that can significantly enhance the performance of your Go applications.