Chapter 10: Real-world Projects and Best Practices with Gin
- With Code Example
- July 21, 2024
Building a RESTful API with Gin: A Step-by-Step Guide
Series - GIN Course
- 1: Chapter-1: Introduction to Gin Framework
- 2: Chapter 2: Setting Up the Environment for GIN Framework
- 3: Chapter 3: Project Structure In Gin Framework
- 4: Chapter 4: Getting Started with Gin
- 5: Chapter 5: Working with Models and Databases
- 6: Chapter 6: Advanced Routing and URL Parameters in Gin
- 7: Chapter 7: Authentication And Authorization In Gin
- 8: Chapter 8: Testing and Debugging in Gin
- 9: Chapter 9: Deploying Gin Applications
- 10: Chapter 10: Real-world Projects and Best Practices with Gin
In this chapter, we’ll delve into building real-world projects with Gin and discuss best practices for developing and maintaining scalable, robust applications. We’ll cover building a RESTful API, explore a case study on building a microservice with Gin, and share essential tips on code organization, error handling, and scaling.
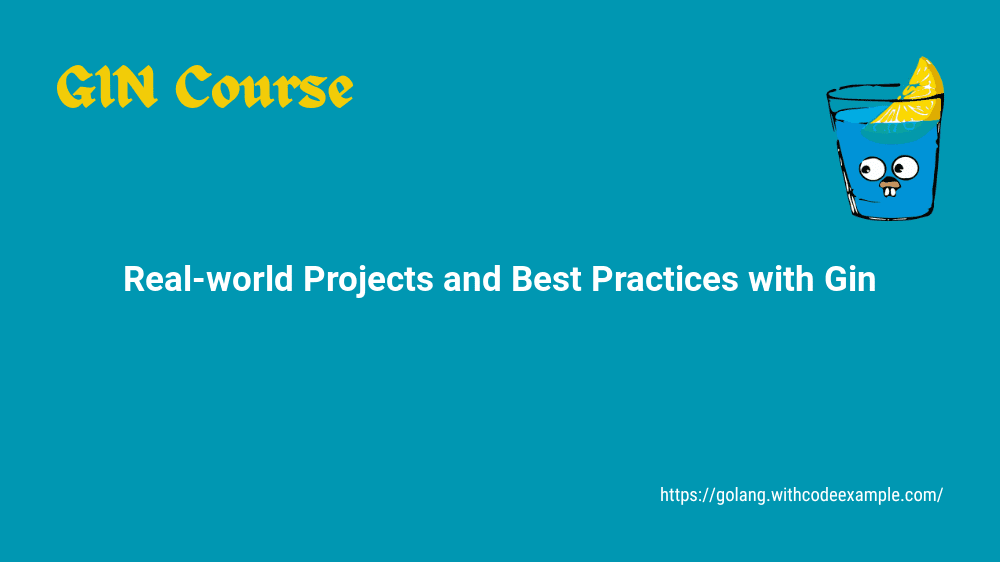
Building a RESTful API with Gin
Designing Endpoints
When building a RESTful API, it’s crucial to design clear, intuitive endpoints that follow standard conventions.
Example: Designing Endpoints
Consider a simple API for managing a collection of books:
- List all books:
GET /books
- Get a specific book:
GET /books/:id
- Create a new book:
POST /books
- Update a book:
PUT /books/:id
- Delete a book:
DELETE /books/:id
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
type Book struct {
ID string `json:"id"`
Title string `json:"title"`
Author string `json:"author"`
}
var books = []Book{
{ID: "1", Title: "1984", Author: "George Orwell"},
{ID: "2", Title: "To Kill a Mockingbird", Author: "Harper Lee"},
}
func main() {
r := gin.Default()
r.GET("/books", getBooks)
r.GET("/books/:id", getBook)
r.POST("/books", createBook)
r.PUT("/books/:id", updateBook)
r.DELETE("/books/:id", deleteBook)
r.Run()
}
func getBooks(c *gin.Context) {
c.JSON(http.StatusOK, books)
}
func getBook(c *gin.Context) {
id := c.Param("id")
for _, book := range books {
if book.ID == id {
c.JSON(http.StatusOK, book)
return
}
}
c.JSON(http.StatusNotFound, gin.H{"message": "book not found"})
}
func createBook(c *gin.Context) {
var newBook Book
if err := c.ShouldBindJSON(&newBook); err != nil {
c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()})
return
}
books = append(books, newBook)
c.JSON(http.StatusCreated, newBook)
}
func updateBook(c *gin.Context) {
id := c.Param("id")
var updatedBook Book
if err := c.ShouldBindJSON(&updatedBook); err != nil {
c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()})
return
}
for i, book := range books {
if book.ID == id {
books[i] = updatedBook
c.JSON(http.StatusOK, updatedBook)
return
}
}
c.JSON(http.StatusNotFound, gin.H{"message": "book not found"})
}
func deleteBook(c *gin.Context) {
id := c.Param("id")
for i, book := range books {
if book.ID == id {
books = append(books[:i], books[i+1:]...)
c.JSON(http.StatusOK, gin.H{"message": "book deleted"})
return
}
}
c.JSON(http.StatusNotFound, gin.H{"message": "book not found"})
}
Best Practices for API Development
- Use Proper HTTP Methods: Use
GET
,POST
,PUT
, andDELETE
appropriately. - Status Codes: Return appropriate HTTP status codes (
200 OK
,201 Created
,400 Bad Request
,404 Not Found
, etc.). - Validation: Validate input data to ensure it meets the required criteria.
- Error Handling: Provide meaningful error messages and handle errors gracefully.
- Versioning: Use API versioning to manage changes without breaking existing clients (
/v1/books
).
Case Study: Building a Microservice with Gin
Microservices Architecture
Microservices architecture involves breaking down an application into smaller, independent services that communicate over a network. Each service focuses on a specific business function.
Example: Microservices Architecture
Consider an e-commerce application with the following microservices:
- User Service: Manages user accounts.
- Product Service: Manages products.
- Order Service: Manages orders.
Communication Between Microservices
Microservices communicate via APIs. Use REST or gRPC for communication and consider using a message broker like RabbitMQ for asynchronous communication.
Example: Product Service
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
type Product struct {
ID string `json:"id"`
Name string `json:"name"`
Price int `json:"price"`
}
var products = []Product{
{ID: "1", Name: "Laptop", Price: 1000},
{ID: "2", Name: "Smartphone", Price: 500},
}
func main() {
r := gin.Default()
r.GET("/products", getProducts)
r.GET("/products/:id", getProduct)
r.POST("/products", createProduct)
r.PUT("/products/:id", updateProduct)
r.DELETE("/products/:id", deleteProduct)
r.Run(":8081")
}
func getProducts(c *gin.Context) {
c.JSON(http.StatusOK, products)
}
func getProduct(c *gin.Context) {
id := c.Param("id")
for _, product := range products {
if product.ID == id {
c.JSON(http.StatusOK, product)
return
}
}
c.JSON(http.StatusNotFound, gin.H{"message": "product not found"})
}
func createProduct(c *gin.Context) {
var newProduct Product
if err := c.ShouldBindJSON(&newProduct); err != nil {
c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()})
return
}
products = append(products, newProduct)
c.JSON(http.StatusCreated, newProduct)
}
func updateProduct(c *gin.Context) {
id := c.Param("id")
var updatedProduct Product
if err := c.ShouldBindJSON(&updatedProduct); err != nil {
c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()})
return
}
for i, product := range products {
if product.ID == id {
products[i] = updatedProduct
c.JSON(http.StatusOK, updatedProduct)
return
}
}
c.JSON(http.StatusNotFound, gin.H{"message": "product not found"})
}
func deleteProduct(c *gin.Context) {
id := c.Param("id")
for i, product := range products {
if product.ID == id {
products = append(products[:i], products[i+1:]...)
c.JSON(http.StatusOK, gin.H{"message": "product deleted"})
return
}
}
c.JSON(http.StatusNotFound, gin.H{"message": "product not found"})
}
Communication Example
The Order Service can communicate with the Product Service to check product availability before creating an order.
// Pseudocode for Order Service
func createOrder(c *gin.Context) {
// Get product ID from request
productID := c.Param("product_id")
// Call Product Service to check availability
resp, err := http.Get("http://localhost:8081/products/" + productID)
if err != nil || resp.StatusCode != http.StatusOK {
c.JSON(http.StatusNotFound, gin.H{"message": "product not found"})
return
}
// Create order logic here
c.JSON(http.StatusCreated, order)
}
Best Practices and Tips
Code Organization
Organize your code into packages to improve readability and maintainability. Use a structure like:
/project
/controllers
/models
/services
/middlewares
main.go
Error Handling and Logging
Proper error handling and logging are crucial for debugging and maintaining your application.
Example: Error Handling
func getBook(c *gin.Context) {
id := c.Param("id")
for _, book := range books {
if book.ID == id {
c.JSON(http.StatusOK, book)
return
}
}
c.JSON(http.StatusNotFound, gin.H{"error": "book not found"})
}
Example: Logging
package main
import (
"github.com/gin-gonic/gin"
"log"
)
func main() {
r := gin.Default()
r.Use(gin.Logger())
r.Use(gin.Recovery())
r.GET("/ping", func(c *gin.Context) {
log.Println("Ping endpoint hit")
c.JSON(200, gin.H{
"message": "pong",
})
})
r.Run()
}
Maintaining and Scaling Gin Applications
- Use Environment Variables: Manage configurations through environment variables.
- Use Docker: Containerize your application for consistent deployment.
- Monitor Performance: Use monitoring tools to keep track of application performance.
- Horizontal Scaling: Deploy multiple instances and use load balancers to distribute traffic.
- Database Optimization: Optimize your database queries and consider using caching mechanisms.
By following the practices outlined in this chapter, you can build, maintain, and scale robust Gin applications. Happy coding!