Chapter 7: Authentication And Authorization In Gin
- With Code Example
- July 21, 2024
Unlocking the Power of Gin: Authentication and Authorization Explained
Series - GIN Course
- 1: Chapter-1: Introduction to Gin Framework
- 2: Chapter 2: Setting Up the Environment for GIN Framework
- 3: Chapter 3: Project Structure In Gin Framework
- 4: Chapter 4: Getting Started with Gin
- 5: Chapter 5: Working with Models and Databases
- 6: Chapter 6: Advanced Routing and URL Parameters in Gin
- 7: Chapter 7: Authentication And Authorization In Gin
- 8: Chapter 8: Testing and Debugging in Gin
- 9: Chapter 9: Deploying Gin Applications
- 10: Chapter 10: Real-world Projects and Best Practices with Gin
In this chapter, we’ll explore the essential aspects of authentication and authorization in the Gin framework. This includes implementing basic and token-based authentication, using role-based access control, applying middleware for authorization, and securing your application with HTTPS and vulnerability prevention.
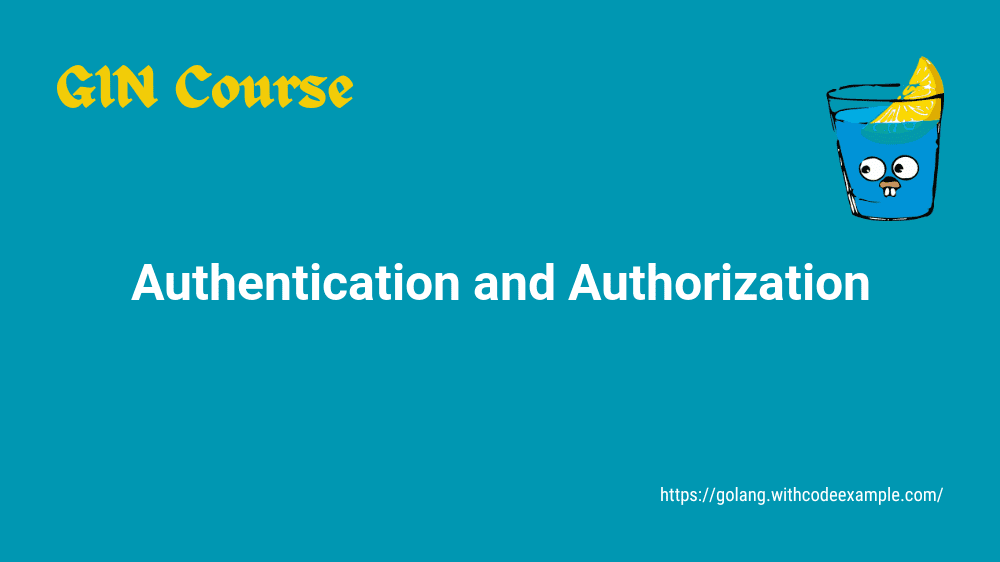
Implementing Authentication
Basic Authentication
Basic authentication is a simple authentication scheme built into the HTTP protocol. It involves sending the username and password with each request. Although it is easy to implement, it’s not recommended for production applications due to security concerns.
Example: Basic Authentication
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
func main() {
r := gin.Default()
authorized := r.Group("/", gin.BasicAuth(gin.Accounts{
"admin": "password123",
}))
authorized.GET("/protected", func(c *gin.Context) {
user := c.MustGet(gin.AuthUserKey).(string)
c.JSON(http.StatusOK, gin.H{"user": user, "message": "Welcome to the protected route!"})
})
r.Run()
}
In this example, the gin.BasicAuth
middleware checks for a valid username and password. If the credentials are correct, the request proceeds; otherwise, it returns a 401 Unauthorized status.
Token-based Authentication (JWT)
Token-based authentication, particularly using JSON Web Tokens (JWT), is a more secure and scalable approach. JWTs are stateless, meaning the server does not need to store session information.
Example: Token-based Authentication
package main
import (
"github.com/gin-gonic/gin"
"github.com/dgrijalva/jwt-go"
"net/http"
"time"
)
var jwtKey = []byte("my_secret_key")
type Claims struct {
Username string `json:"username"`
jwt.StandardClaims
}
func main() {
r := gin.Default()
r.POST("/login", login)
r.GET("/protected", authenticateJWT(), protected)
r.Run()
}
func login(c *gin.Context) {
var creds struct {
Username string `json:"username"`
Password string `json:"password"`
}
if err := c.ShouldBindJSON(&creds); err != nil {
c.JSON(http.StatusBadRequest, gin.H{"error": "Invalid request"})
return
}
if creds.Username == "admin" && creds.Password == "password123" {
expirationTime := time.Now().Add(5 * time.Minute)
claims := &Claims{
Username: creds.Username,
StandardClaims: jwt.StandardClaims{
ExpiresAt: expirationTime.Unix(),
},
}
token := jwt.NewWithClaims(jwt.SigningMethodHS256, claims)
tokenString, err := token.SignedString(jwtKey)
if err != nil {
c.JSON(http.StatusInternalServerError, gin.H{"error": "Could not generate token"})
return
}
c.JSON(http.StatusOK, gin.H{"token": tokenString})
} else {
c.JSON(http.StatusUnauthorized, gin.H{"error": "Invalid credentials"})
}
}
func authenticateJWT() gin.HandlerFunc {
return func(c *gin.Context) {
tokenString := c.GetHeader("Authorization")
claims := &Claims{}
token, err := jwt.ParseWithClaims(tokenString, claims, func(token *jwt.Token) (interface{}, error) {
return jwtKey, nil
})
if err != nil || !token.Valid {
c.JSON(http.StatusUnauthorized, gin.H{"error": "Invalid token"})
c.Abort()
return
}
c.Set("username", claims.Username)
c.Next()
}
}
func protected(c *gin.Context) {
username := c.MustGet("username").(string)
c.JSON(http.StatusOK, gin.H{"message": "Welcome to the protected route!", "user": username})
}
In this example, the login
endpoint generates a JWT for valid credentials, and the authenticateJWT
middleware checks the token’s validity.
Authorization Techniques
Role-based Access Control
Role-based access control (RBAC) restricts access based on the roles assigned to users. This method is effective in managing permissions across different levels of users.
Example: Role-based Access Control
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
func main() {
r := gin.Default()
admin := r.Group("/admin", roleMiddleware("admin"))
admin.GET("/dashboard", adminDashboard)
r.Run()
}
func roleMiddleware(role string) gin.HandlerFunc {
return func(c *gin.Context) {
userRole := c.GetHeader("Role")
if userRole != role {
c.JSON(http.StatusForbidden, gin.H{"error": "Access forbidden"})
c.Abort()
return
}
c.Next()
}
}
func adminDashboard(c *gin.Context) {
c.JSON(http.StatusOK, gin.H{"message": "Welcome to the admin dashboard!"})
}
In this example, the roleMiddleware
checks if the user has the appropriate role to access the route.
Middleware for Authorization
Middleware functions can be used to handle authorization logic centrally, making it easier to manage access control across the application.
Example: Middleware for Authorization
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
func main() {
r := gin.Default()
r.Use(authMiddleware)
r.GET("/profile", userProfile)
r.Run()
}
func authMiddleware(c *gin.Context) {
token := c.GetHeader("Authorization")
if token != "valid-token" {
c.JSON(http.StatusUnauthorized, gin.H{"error": "Unauthorized"})
c.Abort()
return
}
c.Next()
}
func userProfile(c *gin.Context) {
c.JSON(http.StatusOK, gin.H{"message": "User profile"})
}
In this example, the authMiddleware
checks if the request has a valid authorization token.
Securing Your Application
HTTPS Setup
Setting up HTTPS is crucial for securing your application by encrypting the data exchanged between the client and the server.
Example: HTTPS Setup
To set up HTTPS, you’ll need a valid SSL certificate. For development purposes, you can use a self-signed certificate.
package main
import (
"github.com/gin-gonic/gin"
"log"
)
func main() {
r := gin.Default()
// Your routes here
// Replace with your certificate and key files
err := r.RunTLS(":443", "server.crt", "server.key")
if err != nil {
log.Fatal("Failed to start server: ", err)
}
}
In this example, the RunTLS
method starts the server with HTTPS using the provided certificate and key files.
Preventing Common Security Vulnerabilities
Preventing SQL Injection
Always use parameterized queries or ORM libraries to prevent SQL injection attacks.
package main
import (
"github.com/gin-gonic/gin"
"net/http"
"database/sql"
_ "github.com/go-sql-driver/mysql"
)
func main() {
r := gin.Default()
db, err := sql.Open("mysql", "user:password@/dbname")
if err != nil {
panic(err)
}
r.GET("/user/:id", func(c *gin.Context) {
id := c.Param("id")
var name string
err := db.QueryRow("SELECT name FROM users WHERE id = ?", id).Scan(&name)
if err != nil {
c.JSON(http.StatusInternalServerError, gin.H{"error": "User not found"})
return
}
c.JSON(http.StatusOK, gin.H{"name": name})
})
r.Run()
}
In this example, the query uses a parameterized statement to prevent SQL injection.
Preventing Cross-Site Scripting (XSS)
Sanitize any user input to prevent XSS attacks. Use a library like bluemonday
to sanitize HTML input.
package main
import (
"github.com/gin-gonic/gin"
"github.com/microcosm-cc/bluemonday"
)
func main() {
r := gin.Default()
r.POST("/comment", func(c *gin.Context) {
comment := c.PostForm("comment")
policy := bluemonday.UGCPolicy()
sanitizedComment := policy.Sanitize(comment)
c.JSON(200, gin.H{"comment": sanitizedComment})
})
r.Run()
}
In this example, user input is sanitized before being used.
By following the practices outlined in this chapter, you can implement robust authentication and authorization mechanisms in your Gin applications, ensuring they are secure and reliable. Happy coding!