Golang Websocket With Example
- With Code Example
- January 9, 2024
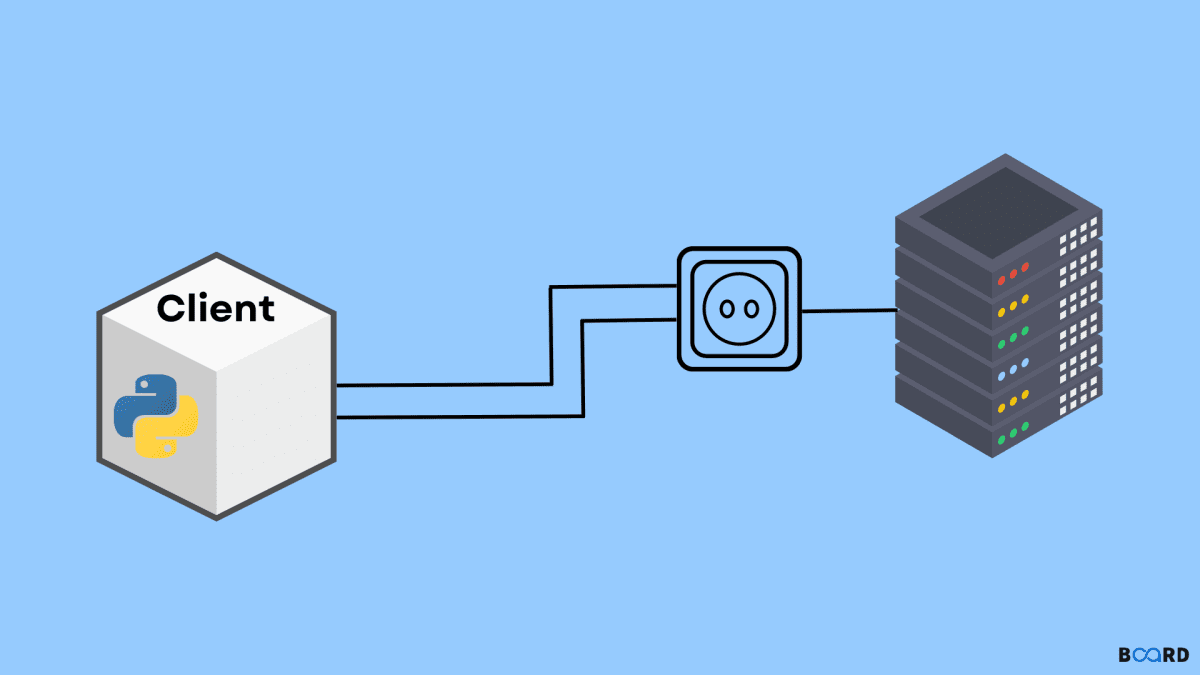
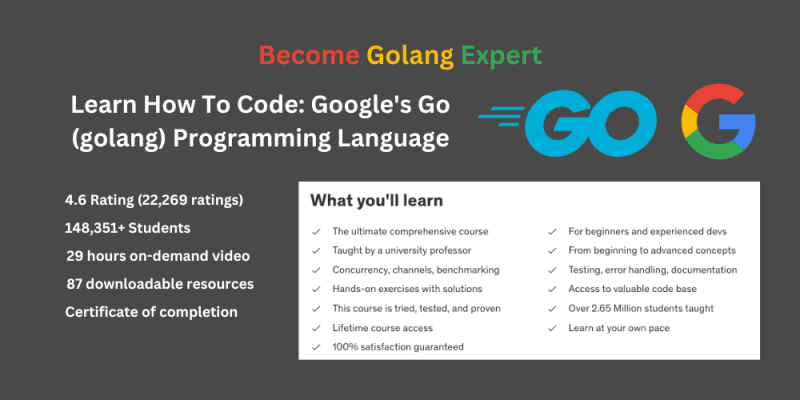
Socket programming, the technique of enabling communication between two nodes in a network, forms the bedrock for constructing sturdy and scalable network applications. Among modern programming languages, Go (also referred to as Golang) has emerged as an exceptional option for socket programming owing to its built-in concurrency constructs. In Go, lightweight threads known as goroutines allow developers to efficiently handle multiple connections and requests in parallel.
In this guide, we will uncover the fundamental concepts every Go developer needs to know for socket programming. To start, we will explain the net package in Go’s standard library, which provides an abstraction over OS-specific socket APIs. We will learn how to set up TCP and UDP sockets for common communication protocols like HTTP and DNS. As we progress, we will explore techniques like multiplexing requests onto goroutine worker pools for high throughput.
A key advantage of using Go for socket programming is unlocking the power of CSP or Communicating Sequential Processes . We will understand how goroutines and channels allow us to tap into the robust parallelism model that Go offers. Practical code snippets will showcase concurrent patterns like pipelines and fan-out/fan-in designs. We will also discuss best practices for aspects like graceful shutdowns and connection pooling.
By the end, you will gain the essential knowledge and hands-on experience with sockets in Go to architect and implement distributed systems, network servers, and cloud-native applications. So let’s get started and master the intricacies of socket programming in Go!
Before starting this tutorial make sure Golang is installed and running on your local machine, If not follow the tutorial to install the Golang on your machine.
Understanding Socket Programming in Go
Socket programming facilitates communication between applications over a network. Go simplifies this process with its rich set of libraries, including the net
and net/http
packages, making it seamless to create sockets and handle network protocols.
Leveraging Go’s Concurrency for Networking
Go’s concurrency model, centred around Goroutines and channels, is a game-changer for network programming. Goroutines, managed by the Go runtime, effortlessly handle thousands of simultaneous connections. Channels facilitate communication between Goroutines, simplifying synchronization complexities and enhancing network application performance.
Want to learn more about Concurrency? Click here
Getting Started: Basic Socket Operations
Let’s start with the basics. In Go, initiating connections and setting up listeners is straightforward with functions like net.Dial
and net.Listen
. The following example showcases a simple TCP server:
package main
import (
"fmt"
"net"
)
func main() {
listener, err := net.Listen("tcp", ":8080")
if err != nil {
fmt.Println("Error:", err)
return
}
defer listener.Close()
for {
conn, err := listener.Accept()
if err != nil {
fmt.Println("Error:", err)
continue
}
go handleConnection(conn)
}
}
func handleConnection(conn net.Conn) {
// Handle connection logic here
fmt.Println("Accepted connection from", conn.RemoteAddr())
defer conn.Close()
// Read and write data on the connection
}
TCP and UDP Socket Communication
Go supports both TCP and UDP protocols. The following example demonstrates a basic UDP server:
package main
import (
"fmt"
"net"
)
func main() {
conn, err := net.ListenPacket("udp", ":8080")
if err != nil {
fmt.Println("Error:", err)
return
}
defer conn.Close()
buffer := make([]byte, 1024)
for {
n, addr, err := conn.ReadFrom(buffer)
if err != nil {
fmt.Println("Error:", err)
continue
}
go handleUDPData(buffer[:n], addr)
}
}
func handleUDPData(data []byte, addr net.Addr) {
// Handle UDP data logic here
fmt.Printf("Received UDP data from %s: %s\n", addr.String(), string(data))
}
Handling Errors and Exceptions
Effective error handling is crucial in network programming. Here’s an example illustrating error handling for a TCP server:
// ... (Previous TCP server code)
func handleConnection(conn net.Conn) {
defer conn.Close()
// Read and write data on the connection
buffer := make([]byte, 1024)
n, err := conn.Read(buffer)
if err != nil {
fmt.Println("Error reading:", err)
return
}
// Process data received
fmt.Printf("Received data: %s\n", string(buffer[:n]))
}
Real-world Examples: Building Network Applications
Now, let’s delve into practical examples. Below is a simple chat server using Go’s concurrency features:
// ... (Previous TCP server code)
func handleConnection(conn net.Conn) {
defer conn.Close()
// Chat logic here
}
Best Practices for Socket Programming in Go
As you explore socket programming in Go, consider best practices for optimizing code, securing applications, and addressing common challenges. Here are a few tips:
- Optimize resource management.
- Prioritize code maintainability.
- Implement secure communication practices.
Conclusion
Socket programming, the fundamental technique for inter-process communication over networks, enables crafting versatile distributed systems in Go. The language’s built-in concurrency constructs make Go a formidable choice for socket programming compared to conventional options. By harnessing the parallelism unlocked through goroutines and channels , Go socket programs can achieve high throughput and low latency while staying resilient.
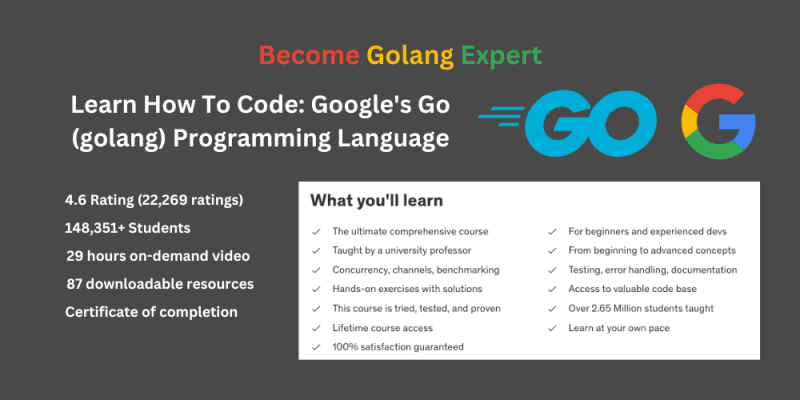
Related Posts
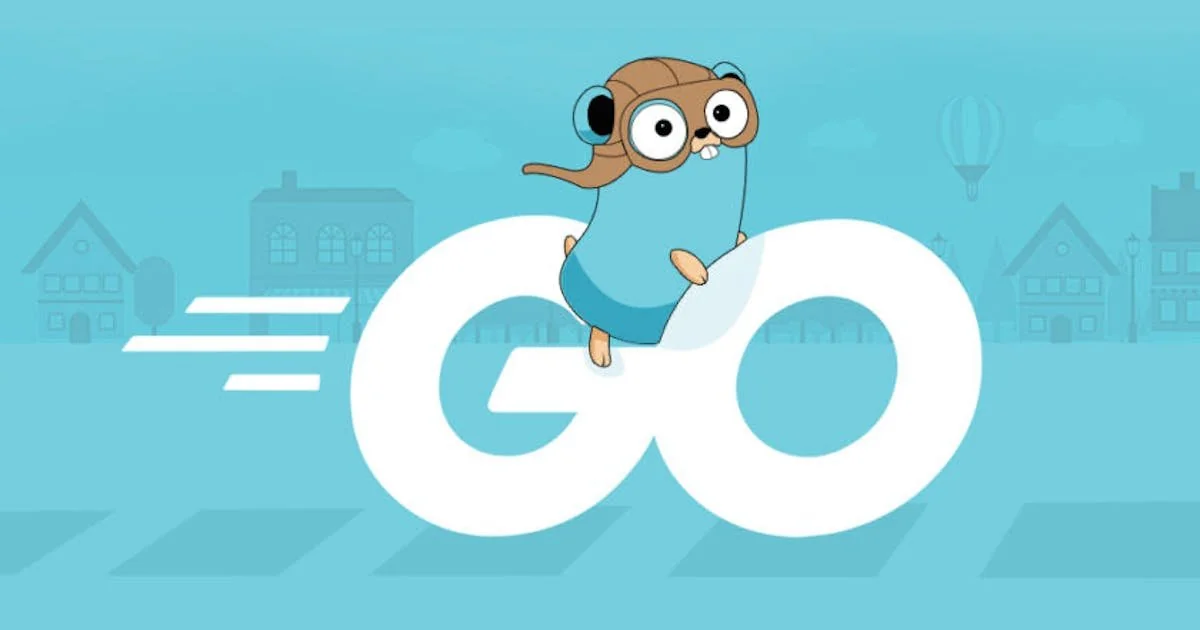
Generics in GoLang: A Guide to Better Code Reuse
Generics have been a much-anticipated feature for Go developers, providing a means to write more flexible and reusable code. Generics allow functions, data structures, and types to operate with any data type while still benefiting from Go’s static typing and performance advantages.