How To Integrate Google Sheets With Golang
- With Code Example
- September 4, 2024
Manage data using the Golang and Google Sheets API.
In today’s data-driven world, the ability to interact with spreadsheets programmatically can be a game-changer. Google Sheets, with its powerful API, offers a fantastic opportunity for developers to automate data processing and analysis. In this article, we’ll explore how to integrate Google Sheets with Golang, enabling you to read, write, and manipulate spreadsheet data effortlessly.
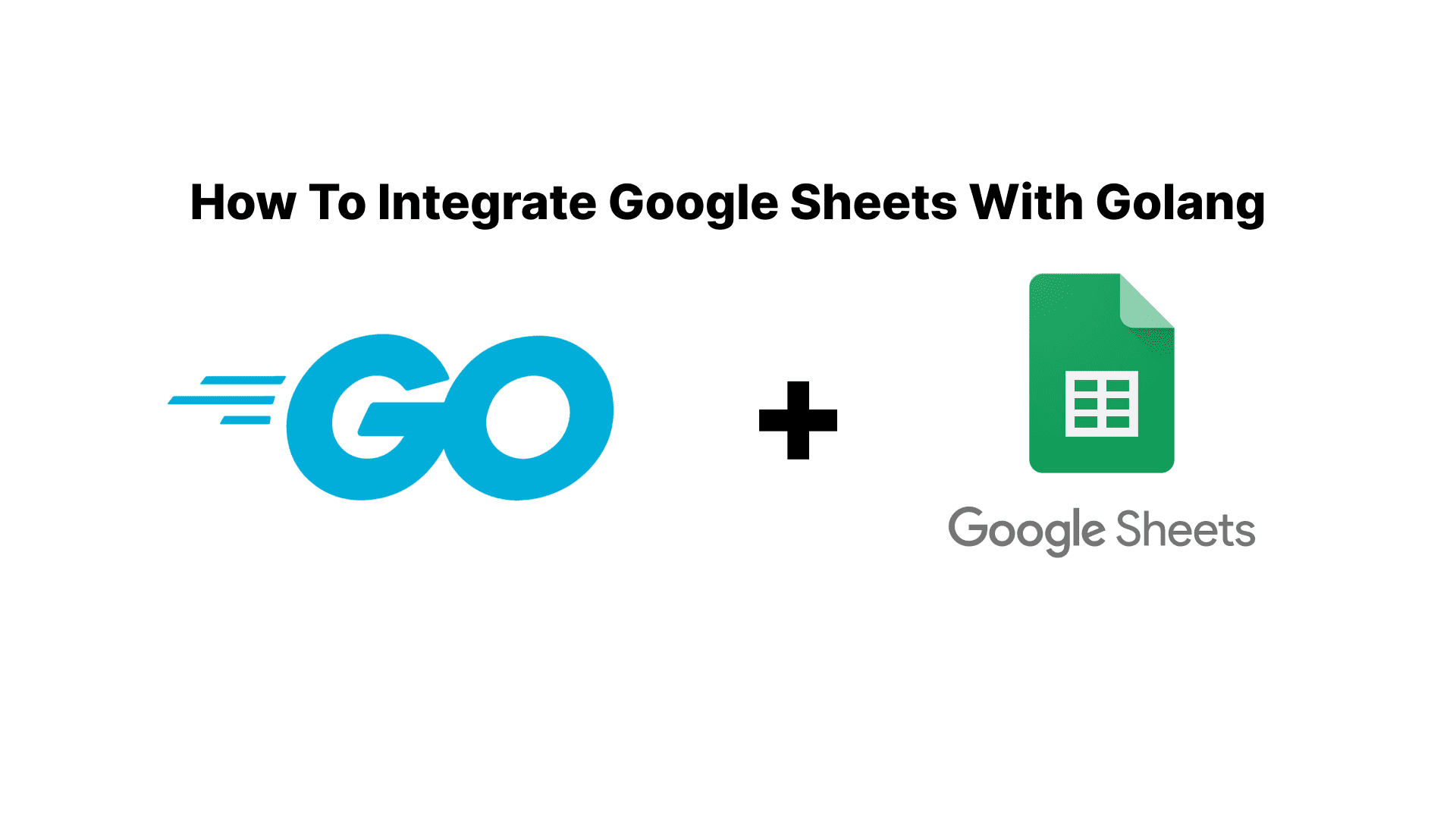
Setting Up Your Golang Google Sheets Project
Before diving into the code, you’ll need to set up your project and obtain the necessary credentials. Let’s walk through this process step-by-step:
Create a new Google Cloud Project
- Go to the Google Cloud Console .
- Click on the project dropdown at the top of the page.
- Click “New Project” and give it a name, then click “Create”.
- Make sure your new project is selected in the dropdown.
Enable the Google Sheets API
- In the Google Cloud Console, navigate to “APIs & Services” > “Library”.
- Search for “Google Sheets API” and click on it.
- Click the “Enable” button.
Create credentials (Service Account Key)
- In the Google Cloud Console, go to “APIs & Services” > “Credentials”.
- Click “Create Credentials” and select “Service Account”.
- Fill in the service account details and click “Create”.
- In the “Service Account Permissions” section, you can skip adding a role for now.
- Click “Continue” and then “Done”.
- Find your new service account in the list, click on it, then go to the “Keys” tab.
- Click “Add Key” > “Create new key”, choose JSON, and click “Create”.
- The key file will be downloaded to your computer. Keep this file secure and don’t share it.
Install the required Golang packages Open your terminal and run the following commands:
go get -u google.golang.org/api/sheets/v4 go get -u golang.org/x/oauth2/google
Set up your Golang project
Create a new directory for your project and navigate into it:
mkdir golang-sheets-project cd golang-sheets-project
Initialize a new Go module:
go mod init myproject
Create a new file called
main.go
. This is where you’ll write your Golang code.
Configure your credentials
Move the JSON key file you downloaded earlier into your project directory.
Set an environment variable to point to this file:
export GOOGLE_APPLICATION_CREDENTIALS="/path/to/your/service-account-key.json"
Alternatively, you can load the file directly in your code, but using an environment variable is generally considered more secure.
Now that you’ve completed these steps, you’re ready to start coding! Your project is set up with the necessary API access and credentials, and you have the required Golang packages installed.
Connecting to Google Sheets
Once you have your credentials, you can establish a connection to the Google Sheets API. Here’s a simple example of how to do this in Golang:
package main
import (
"context"
"log"
"golang.org/x/oauth2/google"
"google.golang.org/api/option"
"google.golang.org/api/sheets/v4"
)
func main() {
ctx := context.Background()
// Load the service account key JSON file
creds, err := google.FindDefaultCredentials(ctx, sheets.SpreadsheetsScope)
if err != nil {
log.Fatalf("Unable to find default credentials: %v", err)
}
// Create a new Sheets service
srv, err := sheets.NewService(ctx, option.WithCredentials(creds))
if err != nil {
log.Fatalf("Unable to create Sheets service: %v", err)
}
log.Println("Successfully connected to Google Sheets API")
}
This code snippet sets up the connection to the Google Sheets API using your service account credentials.
Reading Data from Google Sheets
Now that we’re connected, let’s read some data from a Google Sheet:
func readSheet(srv *sheets.Service, spreadsheetId string, readRange string) {
resp, err := srv.Spreadsheets.Values.Get(spreadsheetId, readRange).Do()
if err != nil {
log.Fatalf("Unable to retrieve data from sheet: %v", err)
}
if len(resp.Values) == 0 {
log.Println("No data found.")
} else {
for _, row := range resp.Values {
// Print columns A and B, which correspond to indices 0 and 1.
log.Printf("%s, %s\n", row[0], row[1])
}
}
}
To use this function, you’d call it like this:
spreadsheetId := "your-spreadsheet-id"
readRange := "Sheet1!A1:B"
readSheet(srv, spreadsheetId, readRange)
Writing Data to Google Sheets
Writing data is just as straightforward. Here’s a function to append rows to a sheet:
func writeToSheet(srv *sheets.Service, spreadsheetId string, writeRange string, values [][]interface{}) {
valueRange := &sheets.ValueRange{
Values: values,
}
_, err := srv.Spreadsheets.Values.Append(spreadsheetId, writeRange, valueRange).ValueInputOption("USER_ENTERED").Do()
if err != nil {
log.Fatalf("Unable to write data to sheet: %v", err)
}
log.Println("Data written successfully")
}
You can use this function like so:
data := [][]interface{}{
{"John Doe", "30", "Software Engineer"},
{"Jane Smith", "28", "Data Analyst"},
}
writeRange := "Sheet1!A1"
writeToSheet(srv, spreadsheetId, writeRange, data)
Putting It All Together
Here’s a complete example that reads from one sheet and writes to another:
package main
import (
"context"
"log"
"golang.org/x/oauth2/google"
"google.golang.org/api/option"
"google.golang.org/api/sheets/v4"
)
func main() {
ctx := context.Background()
creds, err := google.FindDefaultCredentials(ctx, sheets.SpreadsheetsScope)
if err != nil {
log.Fatalf("Unable to find default credentials: %v", err)
}
srv, err := sheets.NewService(ctx, option.WithCredentials(creds))
if err != nil {
log.Fatalf("Unable to create Sheets service: %v", err)
}
spreadsheetId := "your-spreadsheet-id"
readRange := "Sheet1!A1:B"
writeRange := "Sheet2!A1"
// Read data
resp, err := srv.Spreadsheets.Values.Get(spreadsheetId, readRange).Do()
if err != nil {
log.Fatalf("Unable to retrieve data from sheet: %v", err)
}
// Write data to another sheet
valueRange := &sheets.ValueRange{
Values: resp.Values,
}
_, err = srv.Spreadsheets.Values.Append(spreadsheetId, writeRange, valueRange).ValueInputOption("USER_ENTERED").Do()
if err != nil {
log.Fatalf("Unable to write data to sheet: %v", err)
}
log.Println("Data successfully read and written")
}
Conclusion
Integrating Google Sheets with Golang opens up a world of possibilities for data manipulation and automation. Whether you’re building a data pipeline, creating a reporting tool, or simply need to interact with spreadsheets programmatically, the combination of Golang and Google Sheets provides a powerful and efficient solution.
Remember to handle errors gracefully, respect API usage limits, and always secure your credentials. Happy coding!