Learn Pagination and Sorting in GORM
- With Code Example
- September 6, 2023
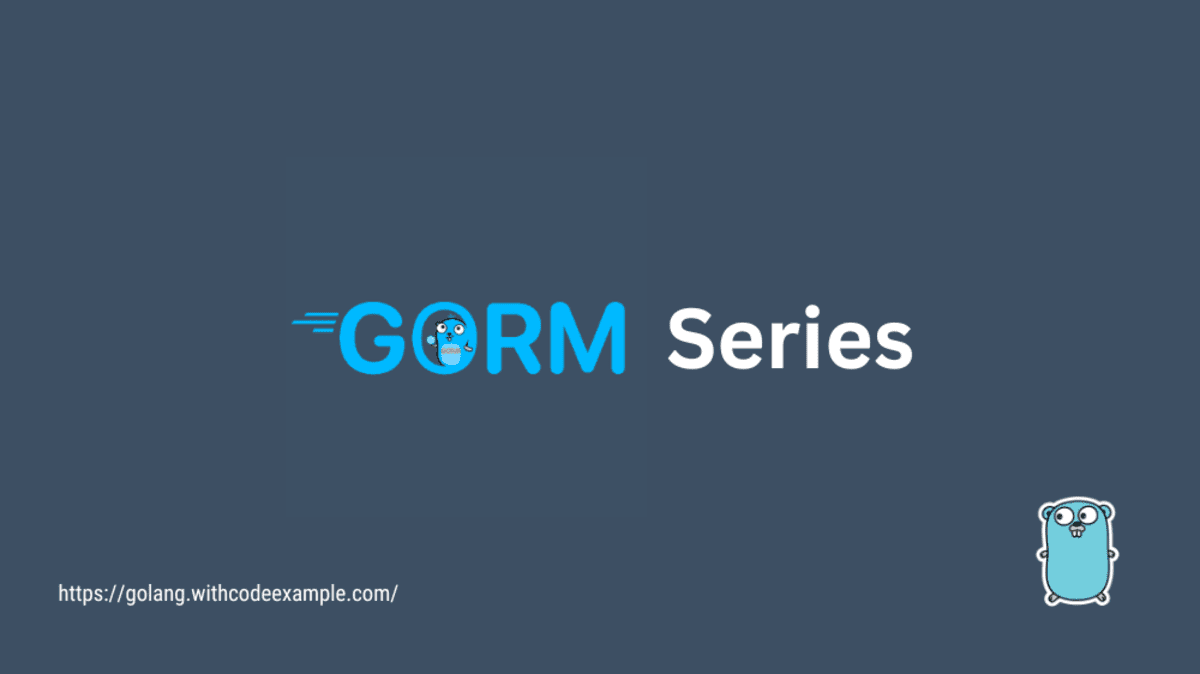
Series - GORM
- 1: GORM: Effortless Database Management in Go
- 2: Defining Models in GORM
- 3: A Guide to CRUD Operations with GORM
- 4: Advanced Querying with GORM
- 5: A Guide to Migrations in GORM
- 6: Transactions and Error Handling with GORM
- 7: Hooks and Callbacks in GORM
- 8: Concurrency and Goroutines in GORM
- 9: Learn Pagination and Sorting in GORM
- 10: Seamlessly Integrating GORM with Go Web Frameworks
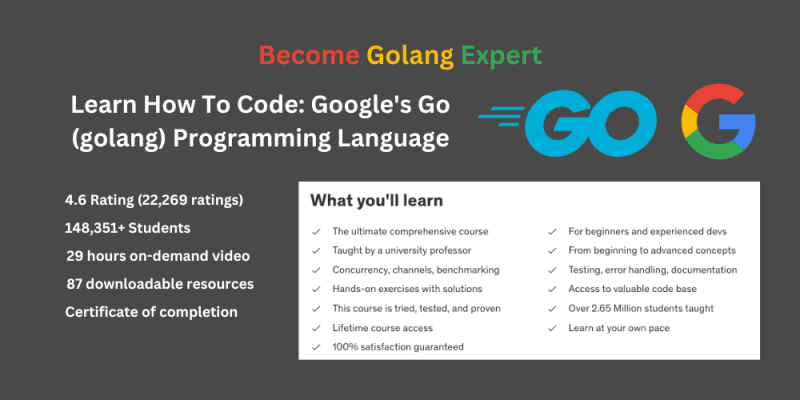
Efficient data retrieval and presentation are crucial aspects of application development. GORM, the robust Go Object-Relational Mapping library, equips developers with powerful tools to achieve this. In this guide, we’ll delve into the world of pagination and sorting in GORM. By the end, you’ll be proficient in implementing these features to streamline data presentation and enhance user experience in your Go projects.
Implementing Pagination with GORM
Pagination enables you to retrieve and present data in manageable chunks, enhancing performance and usability.
Step 1: Limit and Offset
Use GORM’s Limit
and Offset
methods to implement pagination:
var products []Product
db.Limit(10).Offset(20).Find(&products)
Step 2: Paginating With Page Number
Implement pagination using page numbers and a fixed number of records per page:
pageNumber := 2
pageSize := 10
var products []Product
db.Limit(pageSize).Offset((pageNumber - 1) * pageSize).Find(&products)
Sorting Query Results with GORM
Sorting query results according to specific criteria enhances data presentation and usability.
Step 1: Sort Query Results
Use GORM’s Order
method to sort query results:
var sortedProducts []Product
db.Order("price desc").Find(&sortedProducts)
Example: Sorting by Multiple Columns with GORM
To sort query results by multiple columns, use a comma-separated list within the Order
method:
var products []Product
db.Order("category asc, price desc").Find(&products)
Conclusion
Pagination and sorting are essential techniques for efficient data presentation in your applications. GORM’s built-in methods for pagination and sorting provide you with the tools to manage large datasets and tailor their presentation to users’ needs. As you apply the insights and examples from this guide, keep in mind that GORM’s pagination and sorting capabilities are designed to enhance user experience and optimize data interactions in your Go projects. Whether you’re building a dynamic web application or a data-intensive service, mastering pagination and sorting in GORM empowers you to provide a seamless and efficient user experience.
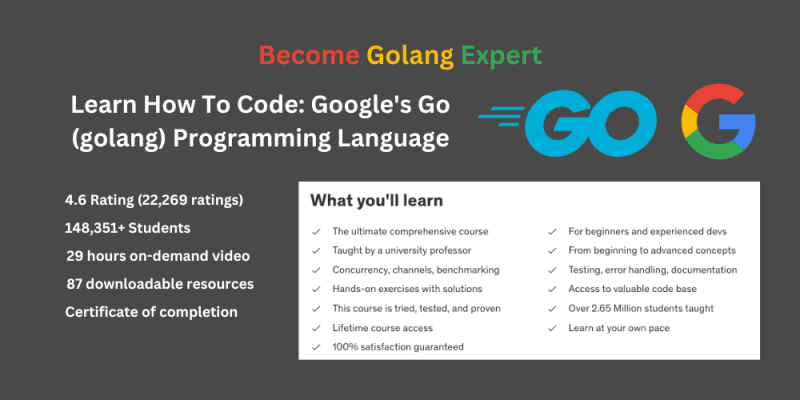
Related Posts
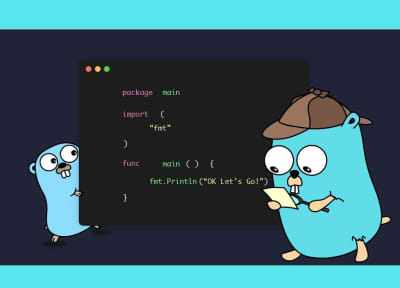
The Art Of Marshal And Unmarshal In Go
Welcome to the complex world of Go programming, where data serialisation and deserialisation take front stage. In the digital symphony of disparate systems, efficient marshalling and unmarshaling emerge as the unsung conductors, allowing for smooth communication, data storage, and dexterous handling of external formats.
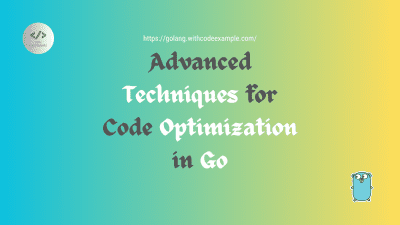
Advanced Techniques for Code Optimization in Go
Go, also known as Golang, is celebrated for its simplicity, readability, and efficiency. While the language itself encourages clean and idiomatic code, there are various advanced techniques and best practices that can significantly enhance the performance of your Go applications.
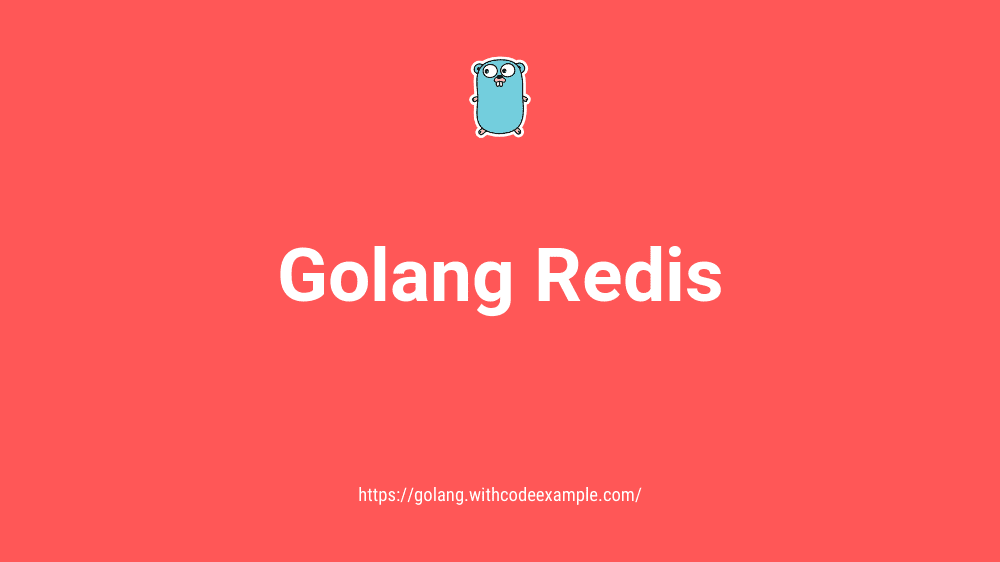
Golang Redis: Building Efficient and Scalable Applications
The necessity for efficient data storage and retrieval has become critical in current application development. Enter Redis, a lightning-fast, open-source, in-memory data structure store that provides a reliable solution for a variety of application scenarios.