Golang Random Number Generation
- With Code Example
- February 8, 2024
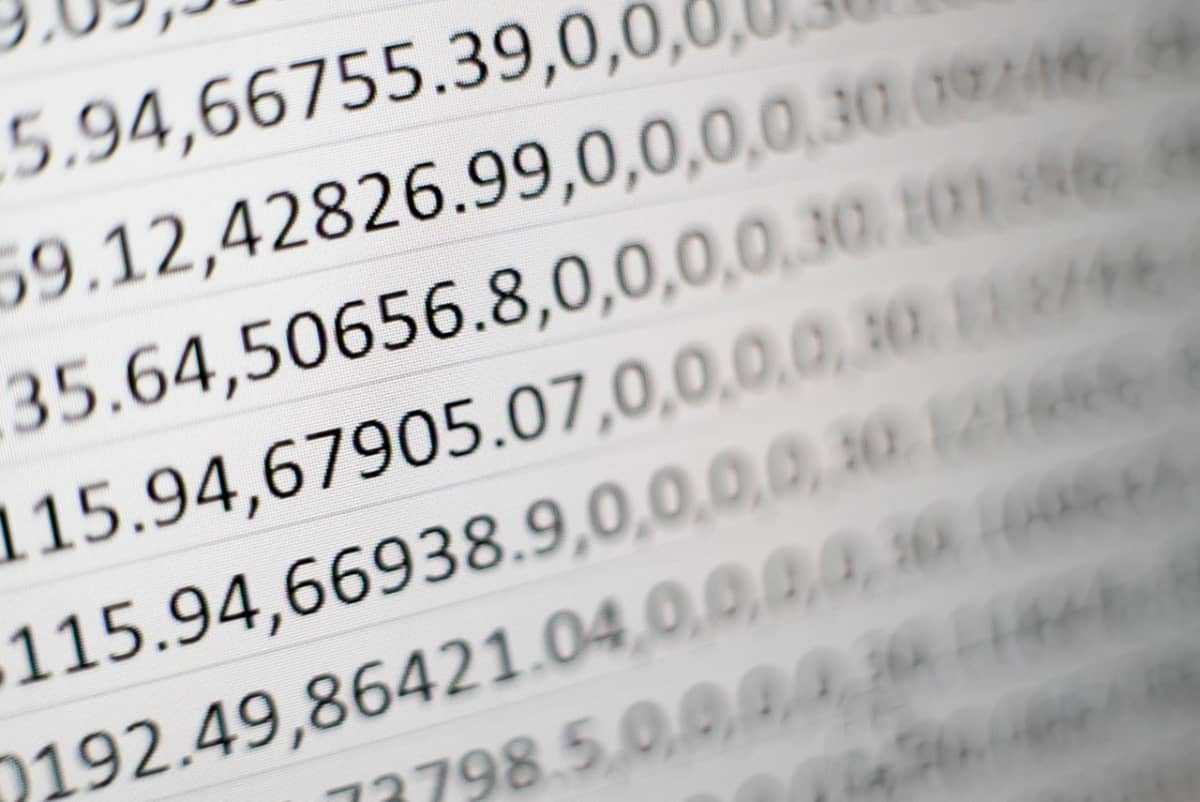
Are you ready to unlock the secrets of Golang random number generation? In the realm of software development, the ability to generate random numbers with precision and efficiency is paramount. From cryptographic applications to simulation algorithms, mastering the intricacies of Golang’s random number generation techniques can elevate your coding prowess to new heights. In this comprehensive guide, we will delve into the depths of Golang’s random number generation algorithms, exploring the nuances of pseudo-randomness, seed values, and distribution functions. Get ready to embark on a journey of discovery as we unravel the mysteries of Golang’s random number generation techniques and empower you to wield them with confidence and finesse.
** Prerequisite** - Golang Tutorial for Beginners a Guide
1. Understanding Golang Random Number Generator:
a. The Basics of Pseudo-Random Numbers:
Before delving into Golang’s specific implementation, it’s essential to understand the concept of pseudo-randomness. Pseudo-random numbers are generated by algorithms that use a seed value to produce a sequence of numbers that appears random. In Golang, the math/rand
package is the primary tool for generating pseudo-random numbers.
b. Seed Values and Reproducibility:
Golang’s random number generator relies on a seed value to initialize the algorithm. The interesting aspect of this is that using the same seed will result in the same sequence of random numbers. While this determinism is beneficial for debugging and testing, it’s crucial to choose seed values carefully to ensure unpredictability in production scenarios.
Run this example here
package main
import (
"fmt"
"math/rand"
"time"
)
func main() {
// Initializing the pseudo-random number generator with a seed based on the current Unix timestamp.
rand.Seed(time.Now().UnixNano())
// Generating and printing a random integer between 0 and 100.
randomNumber := rand.Intn(101)
fmt.Println("Random Number:", randomNumber)
}
2. The math/rand Package in Golang:
a. Basic Usage and Initialization:
Golang’s math/rand
package provides a straightforward API for generating pseudo-random numbers. The first step is to initialize the generator with a seed value using the Seed
function. Once initialized, you can use functions like Intn
to generate random integers within a specified range and Float64
for random floating-point numbers between 0.0 and 1.0.
b. Seeding Best Practices:
Choosing an appropriate seed value is critical for the randomness of the generated sequence. Commonly used methods for seeding include using the current Unix timestamp or incorporating runtime information. It’s advisable to avoid using a constant seed, as this can lead to predictable sequences that compromise the unpredictability of the generated numbers.
Run this example here
package main
import (
"fmt"
"math/rand"
"time"
)
func main() {
// Initializing the pseudo-random number generator with a seed based on the current Unix timestamp.
rand.Seed(time.Now().UnixNano())
// Generating and printing a random integer between 0 and 100.
randomNumber := rand.Intn(101)
fmt.Println("Random Number:", randomNumber)
}
3. Cryptographic Randomness in Golang:
a. Introduction to crypto/rand Package:
For scenarios where higher security and cryptographic randomness are paramount, Golang provides the crypto/rand
package. Unlike math/rand
, which is suitable for most applications, crypto/rand
is designed to provide a more secure source of random numbers.
b. Generating Cryptographically Secure Random Numbers:
The crypto/rand
package introduces the Read
function, allowing the generation of random bytes. These random bytes can then be converted into integers or other data types as needed. It’s crucial to note that cryptographic randomness comes at the cost of performance, so it should be reserved for situations where security is a top priority.
package main
import (
"crypto/rand"
"encoding/binary"
"fmt"
)
func secureRandomInt() (int, error) {
var randomBytes [8]byte
_, err := rand.Read(randomBytes[:])
if err != nil {
return 0, err
}
randomNumber := int(binary.BigEndian.Uint64(randomBytes[:]))
return randomNumber, nil
}
func main() {
secureNumber, err := secureRandomInt()
if err != nil {
fmt.Println("Error generating secure random number:", err)
return
}
fmt.Println("Secure Random Number:", secureNumber)
}
4. Common Pitfalls and Best Practices:
a. Avoiding Common Mistakes:
When working with Golang’s random number generator, there are common pitfalls that developers should be aware of. One such pitfall is the misuse of seed values, leading to predictable sequences. Additionally, relying on math/rand
for cryptographic purposes is a mistake, as it lacks the necessary properties to ensure true randomness in security-sensitive applications.
b. Concurrency Considerations:
Golang’s math/rand
is not designed to be safe for concurrent use by multiple goroutines. Developers must take precautions, such as using locks or providing each goroutine with its own generator, to prevent data races and ensure the integrity of the generated random numbers.
package main
import (
"fmt"
"math/rand"
"sync"
"time"
)
var mu sync.Mutex
func concurrentRandom() int {
mu.Lock()
defer mu.Unlock()
// Ensure that each goroutine gets a unique seed based on the current Unix timestamp.
rand.Seed(time.Now().UnixNano())
return rand.Intn(101)
}
func main() {
var wg sync.WaitGroup
for i := 0; i < 5; i++ {
wg.Add(1)
go func() {
defer wg.Done()
fmt.Println("Concurrent Random Number:", concurrentRandom())
}()
}
wg.Wait()
}
Conclusion:
In conclusion, Golang’s random number generator, anchored by the math/rand
and crypto/rand
packages, offers developers a powerful toolset for generating pseudo-random and cryptographically secure numbers. Understanding the basics of pseudo-randomness, utilizing the math/rand
package for general applications, and employing the crypto/rand
package for cryptographic scenarios are key elements in harnessing the capabilities of Golang’s random number generation.
As you embark on your journey of leveraging Golang’s random number generator, consider the nuances discussed in this guide. Whether you are creating dynamic game experiences, conducting simulations, or ensuring cryptographic security, Golang provides the tools you need.
What are your experiences with Golang’s random number generator? Have you encountered any challenges or discovered unique use cases? Share your insights and join the conversation in the comments below. Your input enriches the community’s knowledge and fosters collaboration.