Chapter 8: Testing and Debugging in Gin
- With Code Example
- July 21, 2024
Ensuring Quality: Testing and Debugging in Gin
Series - GIN Course
- 1: Chapter-1: Introduction to Gin Framework
- 2: Chapter 2: Setting Up the Environment for GIN Framework
- 3: Chapter 3: Project Structure In Gin Framework
- 4: Chapter 4: Getting Started with Gin
- 5: Chapter 5: Working with Models and Databases
- 6: Chapter 6: Advanced Routing and URL Parameters in Gin
- 7: Chapter 7: Authentication And Authorization In Gin
- 8: Chapter 8: Testing and Debugging in Gin
- 9: Chapter 9: Deploying Gin Applications
- 10: Chapter 10: Real-world Projects and Best Practices with Gin
In this chapter, we’ll explore how to write unit tests for Gin applications, use effective debugging techniques, and optimize performance. This includes setting up a testing environment, writing tests for handlers and middleware, using logging, employing debugging tools, and profiling your application for performance improvements.
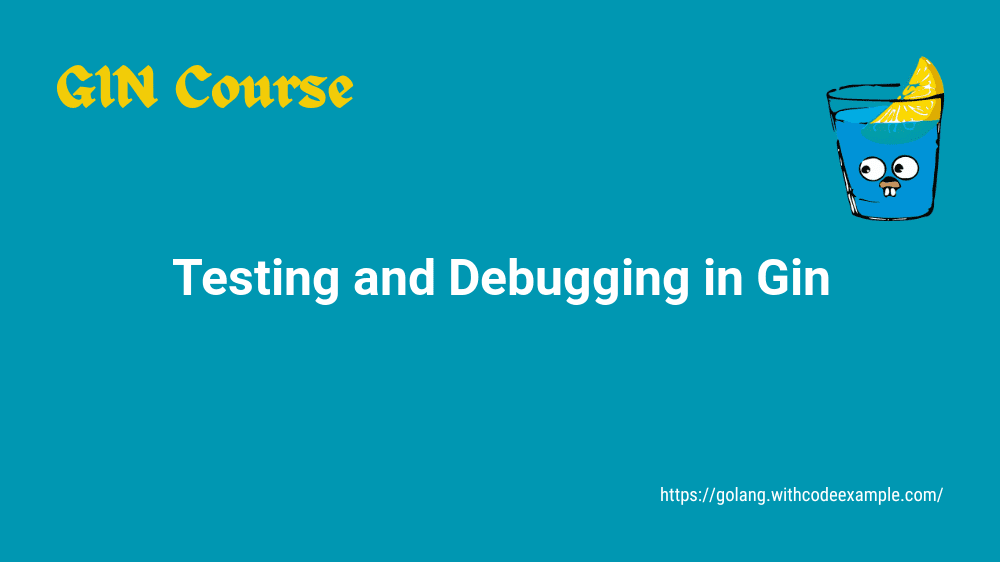
Writing Unit Tests for Gin Applications
Setting up the Testing Environment
Before writing tests, you need to set up your testing environment. This typically involves creating a test file and importing the necessary packages.
Example: Setting up the Testing Environment
Create a file named main_test.go
:
package main
import (
"net/http"
"net/http/httptest"
"testing"
"github.com/gin-gonic/gin"
"github.com/stretchr/testify/assert"
)
func TestMain(m *testing.M) {
gin.SetMode(gin.TestMode)
m.Run()
}
In this setup, we set the Gin mode to TestMode
to reduce the noise during testing.
Writing Tests for Handlers and Middleware
Testing handlers and middleware involves creating a mock HTTP request and response, then asserting the expected outcomes.
Example: Writing Tests for Handlers
Let’s test a simple handler that returns a JSON response.
func TestGetUser(t *testing.T) {
router := gin.Default()
router.GET("/user/:id", getUser)
w := httptest.NewRecorder()
req, _ := http.NewRequest("GET", "/user/123", nil)
router.ServeHTTP(w, req)
assert.Equal(t, http.StatusOK, w.Code)
assert.JSONEq(t, `{"user_id":"123"}`, w.Body.String())
}
In this test, we create a request to the /user/123
endpoint, capture the response, and assert that the status code is 200 OK
and the JSON response matches the expected output.
Example: Writing Tests for Middleware
Testing middleware involves ensuring that it correctly modifies the request/response or blocks the request as expected.
func TestAuthMiddleware(t *testing.T) {
router := gin.Default()
router.Use(AuthMiddleware())
router.GET("/protected", func(c *gin.Context) {
c.JSON(http.StatusOK, gin.H{"message": "Success"})
})
w := httptest.NewRecorder()
req, _ := http.NewRequest("GET", "/protected", nil)
req.Header.Set("Authorization", "Bearer token")
router.ServeHTTP(w, req)
assert.Equal(t, http.StatusOK, w.Code)
assert.JSONEq(t, `{"message":"Success"}`, w.Body.String())
}
In this test, we ensure that the middleware correctly allows the request through when the proper authorization header is set.
Debugging Techniques
Using Logging
Logging is crucial for debugging and understanding the flow of your application. Gin provides built-in support for logging.
Example: Using Logging
package main
import (
"github.com/gin-gonic/gin"
"log"
)
func main() {
r := gin.Default()
r.Use(gin.Logger())
r.Use(gin.Recovery())
r.GET("/ping", func(c *gin.Context) {
log.Println("Ping endpoint hit")
c.JSON(200, gin.H{
"message": "pong",
})
})
r.Run()
}
In this example, gin.Logger()
and gin.Recovery()
are used to log requests and recover from any panics, logging the stack trace.
Debugging Tools and Tips
Using Delve
Delve is a powerful debugger for Go. It allows you to set breakpoints, inspect variables, and control the execution of your application.
- Install Delve:
go install github.com/go-delve/delve/cmd/dlv@latest
- Start Delve:
dlv debug
- Set breakpoints and start debugging:
break main.main
continue
Tips for Effective Debugging
- Reproduce the Issue: Ensure you can consistently reproduce the issue before starting to debug.
- Use Print Statements: Sometimes, simple print statements (
fmt.Println
) can help you understand what’s going on. - Check Logs: Look at your application logs for any clues or errors.
- Divide and Conquer: Narrow down the problematic area by isolating parts of your code.
Performance Optimization
Profiling Your Application
Profiling helps you understand where your application spends most of its time and identifies potential bottlenecks. The Go pprof
package is excellent for profiling.
Example: Profiling with pprof
- Import the
pprof
package and register the handlers:
import (
"net/http"
_ "net/http/pprof"
"github.com/gin-gonic/gin"
)
func main() {
go func() {
log.Println(http.ListenAndServe("localhost:6060", nil))
}()
r := gin.Default()
r.GET("/ping", func(c *gin.Context) {
c.JSON(200, gin.H{
"message": "pong",
})
})
r.Run()
}
- Run your application and then navigate to
http://localhost:6060/debug/pprof/
to see the profiling data.
Improving Performance with Gin
Example: Using Gin’s Built-in Performance Features
- Context Reuse: Reuse contexts to avoid allocations.
package main
import (
"github.com/gin-gonic/gin"
"sync"
)
var pool = sync.Pool{
New: func() interface{} {
return &gin.Context{}
},
}
func main() {
r := gin.Default()
r.GET("/ping", func(c *gin.Context) {
ctx := pool.Get().(*gin.Context)
defer pool.Put(ctx)
ctx.JSON(200, gin.H{
"message": "pong",
})
})
r.Run()
}
Use Appropriate Middleware: Only use necessary middleware to reduce overhead.
Optimize Handlers: Ensure your handlers are efficient and do not perform unnecessary work.
Load Balancing: Use load balancing to distribute the traffic and reduce the load on individual servers.
By following the practices outlined in this chapter, you can write robust unit tests, effectively debug issues, and optimize the performance of your Gin applications. Happy coding!