Building a Golang Gin API with Google OAuth
- With Code Example
- May 3, 2024
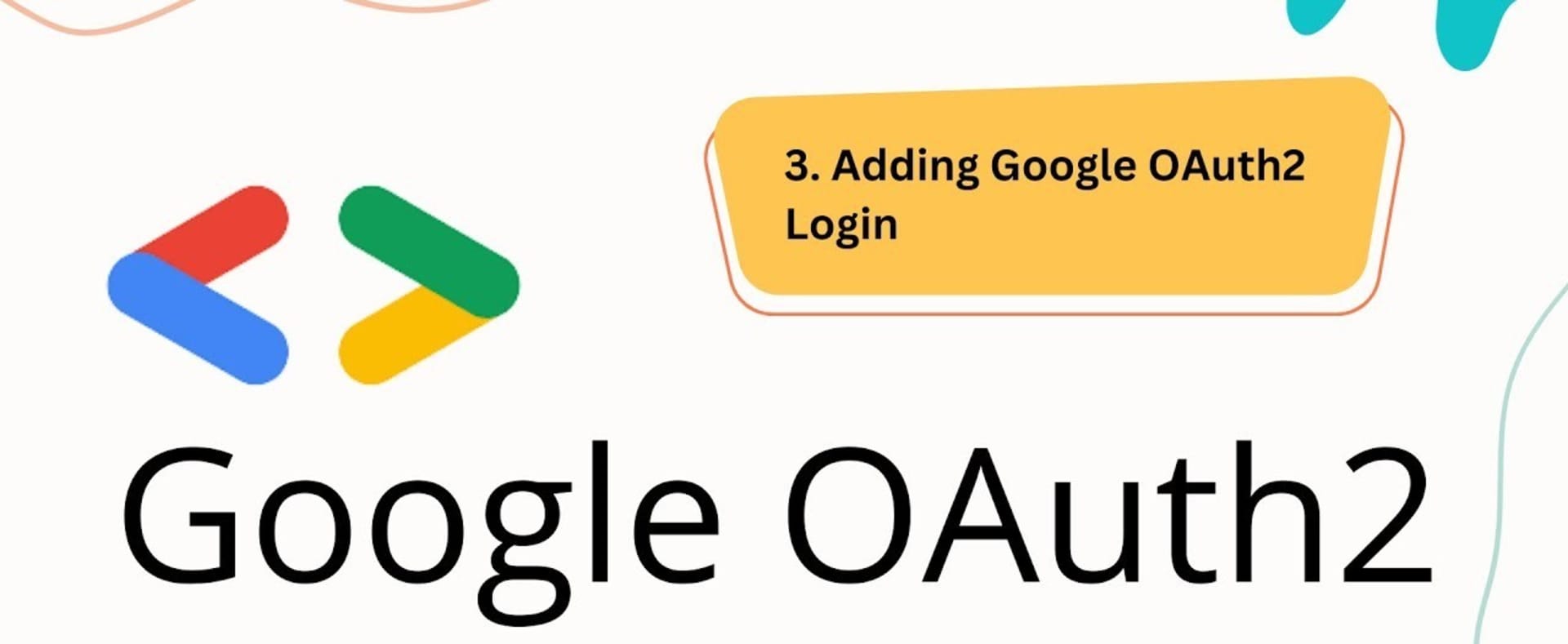
Hello! In today’s article, we will explore how to use Google OAuth in your Gin application. To achieve this, we’ll leverage the github.com/markbates/goth
package, which simplifies OAuth implementation for various providers. Notably, this package has garnered over 5,000 stars on GitHub. Before diving into the setup, I recommend taking a look at the package’s GitHub repository - github.com/markbates/goth
Prerequisites
- Google Oauth Credentials (client_id and client_secret), follow the steps if you don’t have.
- Golang application with gin - follow the steps to create one if you don’t have
Install goth
To start using the goth
in our golan application you have to install it first by running the following command in your application.
go get github.com/markbates/goth
Define Routes
We need to define the two routes for google oauth with gin, first to begain the Oauth and secons is to handle the callback response such as user information and tokens.
// start the google oauth
api.GET("/auth/google/start", controllers.BeginGoogleAuth)
// handle the call back
api.GET("auth/google/callback", controllers.OAuthCallback)
Define the configuration
Then you have to define the configuration for your oauth, you have to use the cient_id
and client_secret
here in this step.
var r = gin.Default()
func init() {
goth.UseProviders(google.New("cleint_id", "client_secret", "http://localhost:8080/auth/google/callback", "email", "profile"))
}
Define start function
func BeginGoogleAuth(c *gin.Context) {
q := c.Request.URL.Query()
q.Add("provider", "google")
c.Request.URL.RawQuery = q.Encode()
gothic.BeginAuthHandler(c.Writer, c.Request)
}
Handle Callback
func OAuthCallback(c *gin.Context) {
q := c.Request.URL.Query()
q.Add("provider", "google")
c.Request.URL.RawQuery = q.Encode()
user, err := gothic.CompleteUserAuth(c.Writer, c.Request)
if err != nil {
c.AbortWithError(http.StatusInternalServerError, err)
return
}
res, err := json.Marshal(user)
if err != nil {
c.AbortWithError(http.StatusInternalServerError, err)
return
}
jsonString := string(res)
c.JSON(http.StatusAccepted, jsonString)
}
Your controller should look like this
package controllers
import (
"encoding/json"
"net/http"
"github.com/gin-gonic/gin"
"github.com/markbates/goth"
"github.com/markbates/goth/gothic"
"github.com/markbates/goth/providers/google"
)
var r = gin.Default()
func init() {
goth.UseProviders(google.New("922329790485-55jrc60i4v62j3n99nqpqbqhesd93on2.apps.googleusercontent.com", "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", "http://localhost:8080/v1/api/auth/google/callback", "email", "profile"))
}
func BeginGoogleAuth(c *gin.Context) {
q := c.Request.URL.Query()
q.Add("provider", "google")
c.Request.URL.RawQuery = q.Encode()
gothic.BeginAuthHandler(c.Writer, c.Request)
}
func OAuthCallback(c *gin.Context) {
q := c.Request.URL.Query()
q.Add("provider", "google")
c.Request.URL.RawQuery = q.Encode()
user, err := gothic.CompleteUserAuth(c.Writer, c.Request)
if err != nil {
c.AbortWithError(http.StatusInternalServerError, err)
return
}
res, err := json.Marshal(user)
if err != nil {
c.AbortWithError(http.StatusInternalServerError, err)
return
}
jsonString := string(res)
c.JSON(http.StatusAccepted, jsonString)
}
A veritable a milestone of Google OAuth into your Gin application is intimately linked up with the perfection of user experience and increased security. Through the utilization of github.com/markbates/goth package you perform two processes simultaneously: simplification of a complicated OAuth process and ensuring the authenticity of your users. With OAuth authentication, an extra security level is added to control access before the user’s Google account details are given to the app without going through the password. This operation, however, reduces errors on their login process while protecting the users’ information and personal data. Besides, the incredible acceptance and trust which goth package shows via its mass usage and high star-rating on GitHub proves that the efficiency this might be.
The strategy is well explained and this coupled with the code examples in this guide, you will be able to easily implement the Google OAuth in the Gin application without any barrier. Weather you’re developing a web application, API, or any other type of service, OAuth authentication with Google deliveres a pan-sphere solution to standardized and secure user access. While adhering to the best possible approaches and keeping the risks of data security in mind, you can be sure that your app is protected with all the ways the users might find enjoyable.
Related Posts
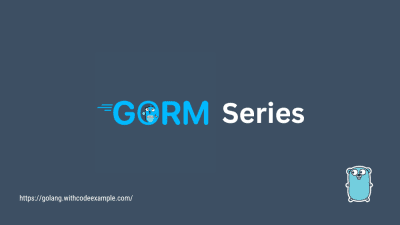
Hooks and Callbacks in GORM
Download PDF In the realm of database management, customization is key to crafting efficient and tailored workflows. GORM, the dynamic Go Object-Relational Mapping library, empowers developers with hooks and callbacks, offering a way to inject custom logic into various stages of the database interaction process.