Advanced Querying with GORM
- With Code Example
- September 1, 2023
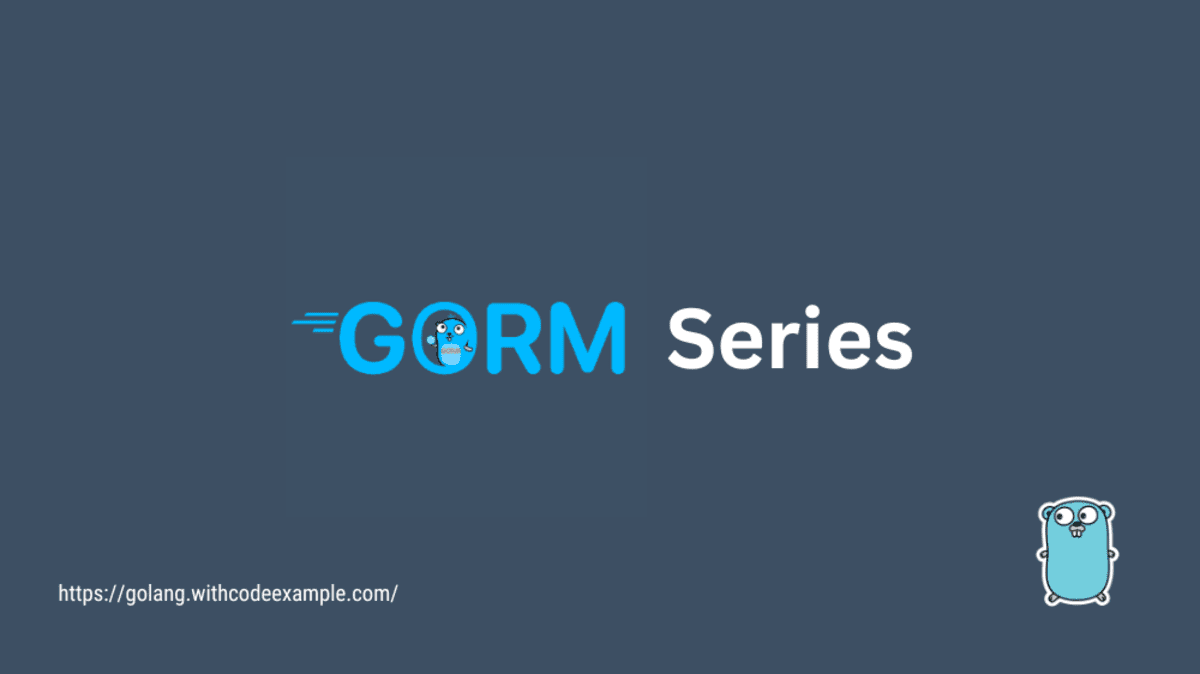
Series - GORM
- 1: GORM: Effortless Database Management in Go
- 2: Defining Models in GORM
- 3: A Guide to CRUD Operations with GORM
- 4: Advanced Querying with GORM
- 5: A Guide to Migrations in GORM
- 6: Transactions and Error Handling with GORM
- 7: Hooks and Callbacks in GORM
- 8: Concurrency and Goroutines in GORM
- 9: Learn Pagination and Sorting in GORM
- 10: Seamlessly Integrating GORM with Go Web Frameworks
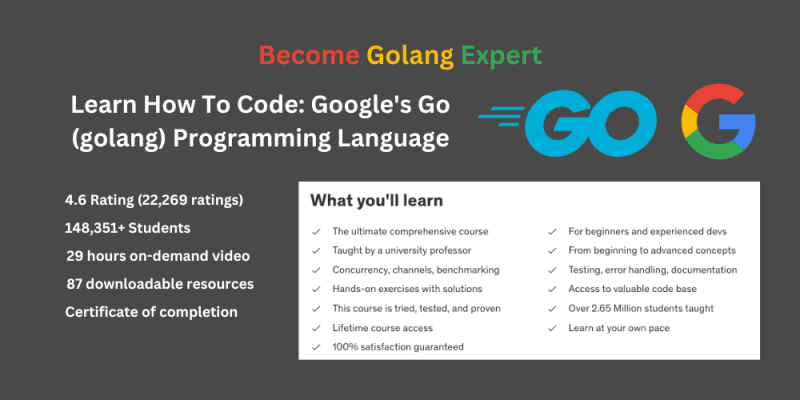
Effective data retrieval is fundamental to the functionality of any programme. The robust Go Object-Relational Mapping package, known as GORM, provides sophisticated querying capabilities in addition to standard CRUD operations. This is your all-in-one resource for learning how to use GORM for advanced queries. WHERE conditions, joins, associations, preloading relevant data, and even dabbling with raw SQL queries will all be covered. By the end, your Go apps will be equipped with the ability to extract and manipulate data with unmatched accuracy.
WHERE Conditions in GORM
Refining queries with WHERE conditions is essential for extracting specific data subsets.
Step 1: Basic WHERE Clause
Use GORM’s Where
method to apply conditions:
var expensiveProducts []Product
db.Where("price > ?", 50).Find(&expensiveProducts)
Step 2: AND & OR Conditions
Combine multiple conditions using logical operators:
var filteredProducts []Product
db.Where("price > ? AND category = ?", 50, "Electronics").Find(&filteredProducts)
Joins and Associations in GORM
Associations between models enable complex queries that span multiple tables.
Step 1: Define Associations
Set up associations in your model structs:
type User struct {
gorm.Model
Orders []Order
}
type Order struct {
gorm.Model
UserID uint
Product string
}
Step 2: Perform Joins
Retrieve data from associated models using GORM’s Joins
method:
var usersWithOrders []User
db.Joins("JOIN orders ON users.id = orders.user_id").Find(&usersWithOrders)
Preloading Related Data in GORM
Efficiently load related data to minimize database queries.
Step 1: Preload Associations
Use GORM’s Preload
method to eagerly load associated data:
var users []User
db.Preload("Orders").Find(&users)
Step 2: Nested Preloading
Preload nested associations for comprehensive data retrieval:
var users []User
db.Preload("Orders.OrderItems").Find(&users)
Raw SQL Queries in GORM
For intricate queries, GORM allows execution of raw SQL statements.
Step 1: Raw SQL Query
Execute raw SQL queries using GORM’s Raw
method:
var products []Product
db.Raw("SELECT * FROM products WHERE price > ?", 50).Scan(&products)
Step 2: Bind Variables
Use bind variables for safer and more efficient queries:
var categoryName = "Electronics"
var expensivePrice = 100
var filteredProducts []Product
db.Raw("SELECT * FROM products WHERE category = ? AND price > ?", categoryName, expensivePrice).Scan(&filteredProducts)
Conclusion
The most comprehensive set of tools for obtaining and modifying data from your Go apps is provided by GORM’s sophisticated querying functionality. You’ve become proficient at precisely and sophisticatedly exploring data by learning how to use joins and relationships, preloading relevant data, and even experimenting with raw SQL queries. These features not only improve the efficiency of your programme but also provide access to previously unthinkable complicated data situations. As you begin your adventure with GORM’s advanced querying, keep in mind that you are the one holding the key to gaining unheard-of control and understanding of the data environment in your application.
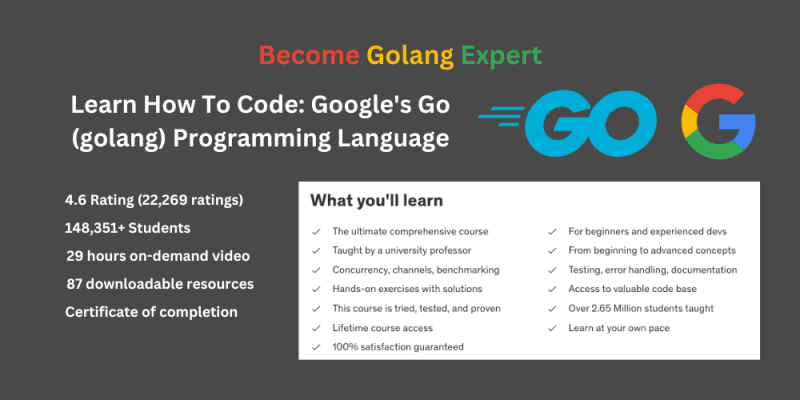